使用unittest框架对线性查找函数进行单元测试
时间: 2024-01-07 19:02:43 浏览: 35
好的,下面是一个简单的线性查找函数示例及其对应的单元测试:
```python
def linear_search(arr, x):
for i in range(len(arr)):
if arr[i] == x:
return i
return -1
import unittest
class TestLinearSearch(unittest.TestCase):
def test_positive_case(self):
arr = [1, 2, 3, 4, 5]
x = 4
self.assertEqual(linear_search(arr, x), 3)
def test_negative_case(self):
arr = [1, 2, 3, 4, 5]
x = 6
self.assertEqual(linear_search(arr, x), -1)
def test_empty_array(self):
arr = []
x = 4
self.assertEqual(linear_search(arr, x), -1)
if __name__ == '__main__':
unittest.main()
```
上面的代码包含3个测试用例:
1. `test_positive_case` 测试了在有序数组 `[1, 2, 3, 4, 5]` 中查找元素 `4`,预期结果是返回索引 `3`。
2. `test_negative_case` 测试了在有序数组 `[1, 2, 3, 4, 5]` 中查找元素 `6`,预期结果是返回 `-1`。
3. `test_empty_array` 测试了在空数组中查找元素 `4`,预期结果是返回 `-1`。
通过使用 `unittest` 框架,我们可以方便地对 `linear_search` 函数进行单元测试,并确保其正确性。
相关推荐
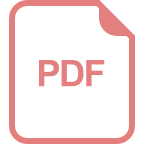
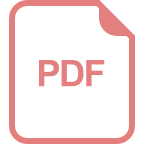














