Levenberg-Marquardt pytorch
时间: 2023-09-02 16:11:49 浏览: 185
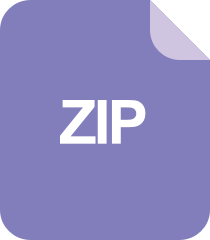
pytorch
Levenberg-Marquardt (LM) is a widely used optimization algorithm for nonlinear least squares problems. In PyTorch, LM can be implemented using the `torch.autograd.functional` module. Here's an example of how to use LM to fit a curve to data:
```python
import torch
from torch.autograd.functional import jacobian
# Define the model
def model(x, params):
a, b, c = params
return a * torch.sin(b * x) + c
# Define the objective function
def objective(params):
y_pred = model(x, params)
residuals = y - y_pred
return torch.sum(residuals ** 2)
# Generate some data
x = torch.linspace(-1, 1, 100)
y = 2 * torch.sin(3 * x) + 0.5 * torch.randn(100)
# Initialize the parameters
params = torch.tensor([1.0, 1.0, 1.0], requires_grad=True)
# Set up the LM optimizer
optimizer = torch.optim.LBFGS([params])
# Train the model
for i in range(100):
def closure():
optimizer.zero_grad()
loss = objective(params)
loss.backward()
return loss
optimizer.step(closure)
# Print the final parameters
print(params)
```
In this example, we define a model that takes an input `x` and a set of parameters `params`, and returns a prediction `y_pred`. We then define an objective function that measures the difference between the predicted values and the actual values (`y` in this case). We generate some random data, initialize the parameters, and set up the LM optimizer. Finally, we train the model by minimizing the objective function using the LM optimizer. The final parameters are printed out.
阅读全文
相关推荐
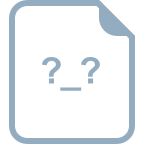


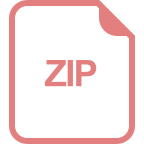
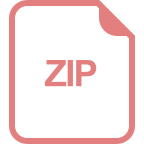
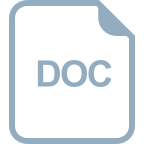



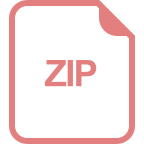
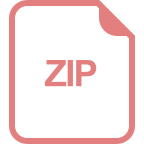