列举一些c#中用到过的设计模式并详细介绍其中一个
时间: 2024-10-09 10:11:16 浏览: 73
C#中常用的一些设计模式包括单例模式、工厂模式、观察者模式、策略模式、模板方法模式、装饰器模式、适配器模式等。其中,我会详细讲解一个常见的例子——工厂模式。
**工厂模式**是一种创建型设计模式,它提供了一种创建对象的最佳方式,而无需暴露其内部细节。这种模式通过一个共同的接口来返回各种具体的产品实例,隐藏了产品具体的生产过程。这种方式使得客户端只需关心如何使用产品,而不必关心产品的具体实现。
例如,在C#中,假设我们要创建一系列交通工具(汽车、飞机、自行车),我们可以有一个抽象的交通工具接口`ITransport`,然后分别有`Car`, `Airplane`, 和 `Bicycle` 类作为具体实现。这时,我们可以创建一个工厂类`TransportFactory`,这个工厂类包含一个静态方法`CreateTransport`,接受一个字符串参数来指示应该创建哪种类型的交通工具:
```csharp
public interface ITransport
{
void Move();
}
public class Car : ITransport
{
public void Move() => Console.WriteLine("开车");
}
public class Airplane : ITransport
{
public void Move() => Console.WriteLine("飞天");
}
public class Bicycle : ITransport
{
public void Move() => Console.WriteLine("骑行");
}
public static class TransportFactory
{
public static ITransport CreateTransport(string type)
{
switch (type)
{
case "car":
return new Car();
case "airplane":
return new Airplane();
case "bicycle":
return new Bicycle();
default:
throw new ArgumentException("Invalid transport type");
}
}
}
```
在这个例子中,客户端只需要传入所需的类型字符串,就可以得到相应的交通工具实例,而不需要了解底层的具体实现。工厂模式有助于模块之间的解耦,并且使得系统更易于扩展。
阅读全文
相关推荐
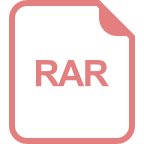
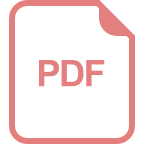
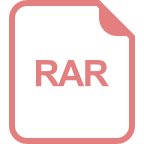
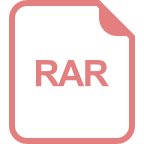
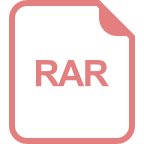
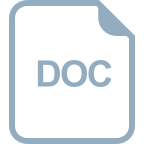
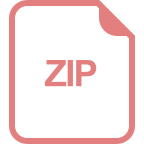
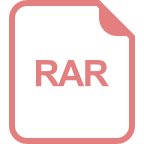
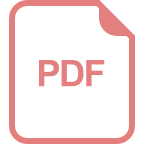
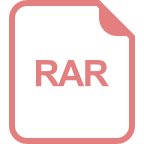
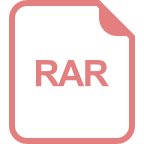
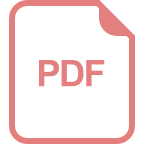
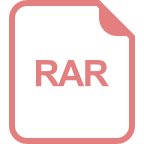
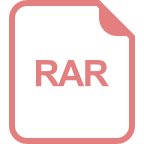
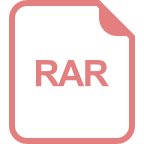