请帮我写一个基于c#+asp.net web的增加试题页面,内容如下:选择学期,科目,题干和选项答案
时间: 2024-05-03 09:19:20 浏览: 12
好的,以下是一个基于C#和ASP.NET Web的增加试题页面示例代码:
首先,您需要在ASP.NET Web应用程序项目中创建一个Web表单。在表单上,您将需要使用ASP.NET Web控件来处理用户输入和数据提交。以下是一个基本的表单布局:
```html
<form id="addQuestionForm" runat="server">
<div>
<label for="semester">选择学期:</label>
<asp:DropDownList ID="semesterDropDownList" runat="server">
<asp:ListItem Text="第一学期" Value="1" />
<asp:ListItem Text="第二学期" Value="2" />
<asp:ListItem Text="第三学期" Value="3" />
</asp:DropDownList>
</div>
<div>
<label for="subject">科目:</label>
<asp:TextBox ID="subjectTextBox" runat="server"></asp:TextBox>
</div>
<div>
<label for="question">题干:</label>
<asp:TextBox ID="questionTextBox" runat="server"></asp:TextBox>
</div>
<div>
<label for="optionA">选项A:</label>
<asp:TextBox ID="optionATextBox" runat="server"></asp:TextBox>
</div>
<div>
<label for="optionB">选项B:</label>
<asp:TextBox ID="optionBTextBox" runat="server"></asp:TextBox>
</div>
<div>
<label for="optionC">选项C:</label>
<asp:TextBox ID="optionCTextBox" runat="server"></asp:TextBox>
</div>
<div>
<label for="optionD">选项D:</label>
<asp:TextBox ID="optionDTextBox" runat="server"></asp:TextBox>
</div>
<div>
<label for="answer">答案:</label>
<asp:DropDownList ID="answerDropDownList" runat="server">
<asp:ListItem Text="A" Value="A" />
<asp:ListItem Text="B" Value="B" />
<asp:ListItem Text="C" Value="C" />
<asp:ListItem Text="D" Value="D" />
</asp:DropDownList>
</div>
<asp:Button ID="submitButton" runat="server" Text="提交" OnClick="submitButton_Click" />
</form>
```
在上面的表单中,我们使用了ASP.NET Web控件DropDownList和TextBox来获取用户输入。我们还添加了一个名为“submitButton”的按钮,当用户单击该按钮时,将调用名为“submitButton_Click”的事件处理程序。
现在,我们需要编写名为“submitButton_Click”的事件处理程序,以便在用户单击提交按钮时将数据保存到数据库中。以下是一个简单的代码示例:
```csharp
protected void submitButton_Click(object sender, EventArgs e)
{
// 获取用户输入的值
string semester = semesterDropDownList.SelectedValue;
string subject = subjectTextBox.Text;
string question = questionTextBox.Text;
string optionA = optionATextBox.Text;
string optionB = optionBTextBox.Text;
string optionC = optionCTextBox.Text;
string optionD = optionDTextBox.Text;
string answer = answerDropDownList.SelectedValue;
// 将数据插入到数据库中
string connectionString = ConfigurationManager.ConnectionStrings["MyConnectionString"].ConnectionString;
using (SqlConnection connection = new SqlConnection(connectionString))
{
string sql = "INSERT INTO Questions (Semester, Subject, Question, OptionA, OptionB, OptionC, OptionD, Answer) VALUES (@Semester, @Subject, @Question, @OptionA, @OptionB, @OptionC, @OptionD, @Answer)";
using (SqlCommand command = new SqlCommand(sql, connection))
{
command.Parameters.AddWithValue("@Semester", semester);
command.Parameters.AddWithValue("@Subject", subject);
command.Parameters.AddWithValue("@Question", question);
command.Parameters.AddWithValue("@OptionA", optionA);
command.Parameters.AddWithValue("@OptionB", optionB);
command.Parameters.AddWithValue("@OptionC", optionC);
command.Parameters.AddWithValue("@OptionD", optionD);
command.Parameters.AddWithValue("@Answer", answer);
connection.Open();
int result = command.ExecuteNonQuery();
if (result > 0)
{
// 提示用户数据已成功保存
Response.Write("数据已成功保存");
}
}
}
}
```
在上面的代码中,我们首先获取用户输入的值,然后将数据插入到名为“Questions”的数据库表中。我们使用了SQL Server数据库,并且假定您已经创建了一个名为“Questions”的表,其中包含学期、科目、问题、选项和答案列。如果插入操作成功,我们将向用户显示一条消息,指示数据已成功保存。
请注意,以上代码仅提供参考。您需要根据自己的需求和数据库架构进行适当的修改。
相关推荐
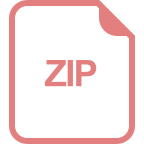
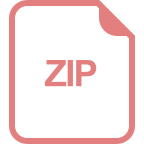
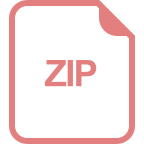














