1、编写一个C程序作为Linux内核的shell命令行解释程序。Shell程序应该使用与Bource shell相同的方式运行程序。 增加功能以使用户可以使用“&”操作符作为命令的结束符。一个以“&”结尾的命令应该与shell并发执行。 增加功能以使用户可以使用“<”和“>”作为文件名前缀来重定向stdin和stdout描述符。同样,允许用户使用管道操作符“|”来同时执行两个进程,并把第一个进程的stdout重定向为第二个进程的stdin。
时间: 2023-12-25 07:05:25 浏览: 77
这是一个相当复杂的任务,需要深入了解Linux内核和系统编程。以下是一个简单的示例程序,它可以解析命令行参数并执行相应的操作。请注意,这个示例程序没有考虑所有可能的情况,实际上需要更多的测试和错误处理。
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/wait.h>
#include <fcntl.h>
#include <string.h>
#define MAX_ARGS 1024
#define MAX_FILENAME 256
int main(int argc, char *argv[]) {
char *args[MAX_ARGS];
char *input_file = NULL;
char *output_file = NULL;
int background = 0;
int pipefd[2];
// Parse command line arguments
int i;
for (i = 1; i < argc; i++) {
if (strcmp(argv[i], "&") == 0) {
background = 1;
} else if (strcmp(argv[i], "<") == 0) {
input_file = argv[++i];
} else if (strcmp(argv[i], ">") == 0) {
output_file = argv[++i];
} else if (strcmp(argv[i], "|") == 0) {
if (pipe(pipefd) == -1) {
perror("pipe");
exit(EXIT_FAILURE);
}
args[i] = NULL;
break;
} else {
args[i-1] = argv[i];
}
}
args[i-1] = NULL;
// Redirect input and/or output
if (input_file != NULL) {
int fd = open(input_file, O_RDONLY);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
dup2(fd, STDIN_FILENO);
close(fd);
}
if (output_file != NULL) {
int fd = open(output_file, O_WRONLY | O_CREAT | O_TRUNC, 0666);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
dup2(fd, STDOUT_FILENO);
close(fd);
}
// Fork and execute command
pid_t pid = fork();
if (pid == -1) {
perror("fork");
exit(EXIT_FAILURE);
} else if (pid == 0) {
if (input_file == NULL && pipefd[0] != 0) {
dup2(pipefd[0], STDIN_FILENO);
close(pipefd[0]);
}
if (output_file == NULL && pipefd[1] != 0) {
dup2(pipefd[1], STDOUT_FILENO);
close(pipefd[1]);
}
execvp(args[0], args);
perror("execvp");
exit(EXIT_FAILURE);
} else {
if (background == 0) {
waitpid(pid, NULL, 0);
}
if (pipefd[1] != 0) {
close(pipefd[1]);
char line[MAX_FILENAME];
while (fgets(line, MAX_FILENAME, stdin) != NULL) {
write(pipefd[1], line, strlen(line));
}
close(pipefd[0]);
}
}
return 0;
}
```
这个程序使用了一些Linux系统编程的概念,包括:
- `fork()`函数:创建一个子进程来执行命令。
- `execvp()`函数:在子进程中执行命令。
- `waitpid()`函数:等待子进程结束。
- 文件描述符和重定向:通过`dup2()`函数将标准输入、标准输出和管道重定向到文件或管道。
- 管道:使用`pipe()`函数创建一个管道,将第一个进程的输出传递给第二个进程的输入。
这个程序还有许多可以改进的地方,例如增加错误处理,支持更多的命令行选项等等。但是,这个简单的示例程序可以帮助你了解如何编写一个基本的shell程序。
阅读全文
相关推荐
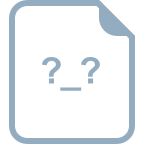
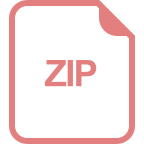



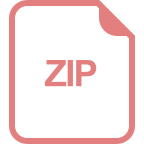
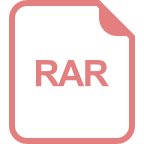
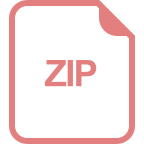
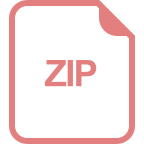
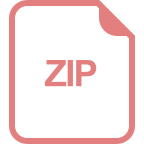
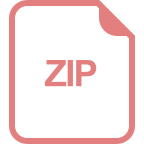
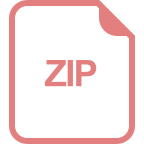
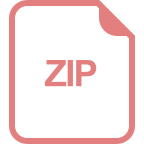
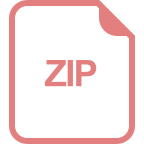
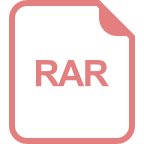
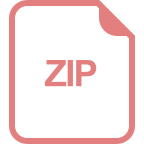