python partial
时间: 2023-10-15 14:25:42 浏览: 80
在 Python 中,`partial` 函数是 `functools` 模块中的一个函数,用于创建一个新的可调用对象,该对象是原函数的部分应用。部分应用是指固定函数的某些参数,以及在调用时只需为剩余的参数提供值。
`partial` 函数的语法如下:
```python
functools.partial(func, *args, **kwargs)
```
其中,`func` 是原函数,`*args` 和 `**kwargs` 是需要部分应用的参数。
使用 `partial` 函数可以方便地创建一个新的函数,该函数在调用时只需提供部分参数即可。例如,假设有以下原函数:
```python
def greet(name, message):
print(f"Hello, {name}! {message}")
```
如果想要创建一个专门用于向某人打招呼的新函数,可以使用 `partial` 函数:
```python
from functools import partial
say_hello = partial(greet, message="How are you?")
say_hello("John")
```
这样,`say_hello` 函数就会打印出 "Hello, John! How are you?"。
通过使用 `partial` 函数,可以更灵活地复用现有函数,并减少在调用时需要提供的参数数量。
相关问题
python partial函数
partial函数是Python标准库functools中的一个函数,它可以用来创建一个新的函数,并将原函数的某些参数固定下来。使用partial函数可以实现函数的柯里化(currying),即将一个接受多个参数的函数转化为一系列只接受部分参数的函数。通过partial函数可以设定函数的默认参数值,从而减少函数调用时需要提供的参数数量。使用partial函数的一种常见场景是在函数式编程中的高阶函数中,通过partial函数可以创建一个新的函数,将原函数的某些参数固定下来,从而生成一个新的函数,这个新函数只接受剩下的参数,并将其传递给原函数进行调用。partial函数的使用方式有两种,一种是使用import导入functools模块,然后通过functools.partial()的方式调用,另一种是直接使用from functools import partial的方式导入partial函数。
python partial, how to assign position of input argument
`functools.partial` in Python allows you to create a new function by partially applying arguments to an existing function. You can also assign the position of the input argument using `functools.partial`.
To assign the position of the input argument, you need to use the `functools.partial` function and pass the argument position as a keyword argument. Here is an example:
```python
import functools
def multiply(x, y):
return x * y
double = functools.partial(multiply, y=2) # Assigns the second argument's position to y
print(double(5)) # Output: 10
```
In the above example, we create a new function `double` from the `multiply` function by partially applying the `y` argument with a value of 2. We also assign the position of the `y` argument as the second argument of `multiply`.
Then, we call the `double` function with `x=5` as the first argument and `y=2` as the second argument. The output is `10`, which is the result of `5 * 2`.
阅读全文
相关推荐
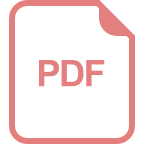
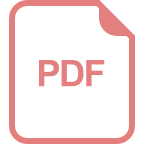
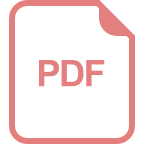





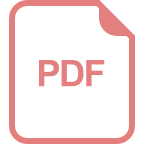
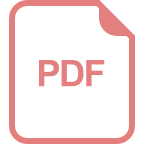
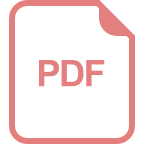
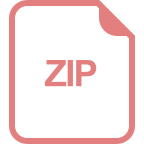
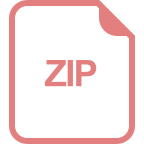
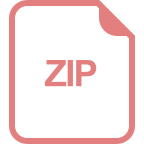
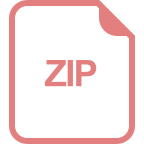
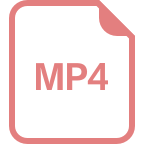