js使用unzipper 递归解压多层的压缩包
时间: 2023-12-02 16:06:27 浏览: 136
要使用 `unzipper` 递归解压多层的压缩包,可以使用递归函数。以下是一个示例代码:
```javascript
const unzipper = require('unzipper');
const fs = require('fs');
async function unzipRecursive(source, target) {
const readStream = fs.createReadStream(source);
const directory = target || source.slice(0, -4);
await readStream
.pipe(unzipper.Parse())
.on('entry', async function (entry) {
const fileName = entry.path;
const type = entry.type; // 'Directory' or 'File'
const fullPath = `${directory}/${fileName}`;
if (type === 'Directory') {
await fs.promises.mkdir(fullPath, { recursive: true });
entry.autodrain();
await unzipRecursive(entry.path, fullPath);
} else {
entry.pipe(fs.createWriteStream(fullPath));
}
})
.promise();
}
```
在上述代码中,`unzipRecursive` 函数接收两个参数:源文件路径和目标目录路径。如果目标目录路径未提供,则默认使用源文件路径去掉扩展名作为目标目录路径。函数中的 `await readStream.pipe(unzipper.Parse())` 表示将源文件流导入 `unzipper` 模块中进行解压,并返回一个解压流。
`unzipper.Parse()` 返回的流会发射 `entry` 事件,表示解压出的每个文件或目录。在 `entry` 事件处理程序中,我们根据 `entry.type` 的值判断当前解压出的是文件还是目录。如果是目录,我们先创建该目录,并递归调用 `unzipRecursive` 函数进行下一级解压;如果是文件,则将该文件写入目标目录。在写入文件时,我们使用 `fs.createWriteStream` 创建一个写入流,并将 `entry` 流导入该写入流中。
需要注意的是,`unzipper` 模块要求每个解压流必须被消耗,否则可能会出现内存泄漏。因此,在处理目录时,我们需要调用 `entry.autodrain()` 完全消耗该流。
阅读全文
相关推荐
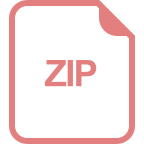
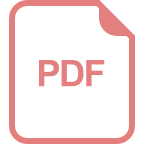


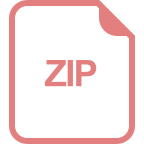
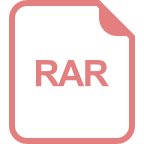
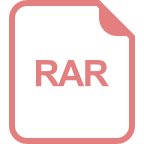
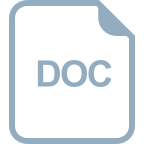

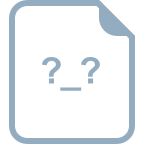
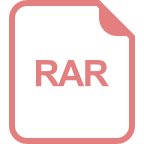
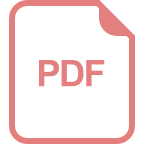
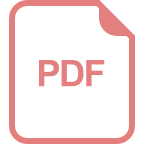
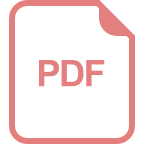
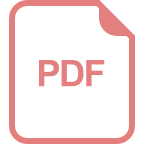

