ValueError: The truth value of an array with more than one element is ambiguous. Use a.any() or a.all().
时间: 2023-10-19 07:23:24 浏览: 22
This error occurs when you try to use an array as a condition in a logical statement, but the array contains more than one element and it is unclear how to interpret the truth value of the array in the context of the logical statement.
To resolve this error, you can use the `.any()` or `.all()` methods on the array to reduce it to a single boolean value that can be used as the condition in the logical statement.
For example, instead of writing:
```
if my_array:
# do something
```
You can write:
```
if my_array.any():
# do something
```
Or:
```
if my_array.all():
# do something
```
Depending on your specific needs and the logic of your code.
相关问题
ValueError: The truth value of an array with more than one element is ambiguous. Use a.any() or a.all(
ValueError: The truth value of an array with more than one element is ambiguous. Use a.any() or a.all() 是一个常见的错误消息,通常在使用NumPy数组进行条件判断时出现。
这个错误的原因是,当你尝试将一个包含多个元素的数组作为条件进行判断时,Python无法确定应该如何解释这个判断。因为一个数组可以包含多个元素,而条件判断通常只能返回一个布尔值。
为了解决这个问题,你可以使用NumPy提供的`any()`或`all()`函数来将数组转换为单个布尔值。这两个函数的作用如下:
- `any()`函数:如果数组中至少有一个元素满足条件,则返回True;否则返回False。
- `all()`函数:只有当数组中的所有元素都满足条件时,才返回True;否则返回False。
你可以根据具体的需求选择使用`any()`或`all()`函数来解决这个错误。
ValueError: The truth value of an array with more than one element is ambiguous.
"ValueError: The truth value of an array with more than one element is ambiguous."这个错误是由于在使用if语句或布尔运算符(如==、!=、<等)时,对于包含多个元素的numpy数组,无法明确地确定其真值造成的。
具体来说,在Python中,对于一个单个元素的变量,可以使用if语句来检查其真值。但是,当变量是一个numpy数组时,由于numpy数组可能包含多个元素,所以无法直接使用if语句来检查其真值。这是因为存在多种可能的解释:数组中的所有元素都为真时,数组中的任意一个元素为真时,或者数组中的所有元素都为假时。因此,需要使用numpy提供的函数来明确指定判断条件,并避免出现歧义。
在这种情况下,可以使用numpy的any()函数或all()函数来判断数组的真值。any()函数用于判断数组中是否存在一个或多个元素为真,而all()函数则用于判断数组中的所有元素是否都为真。通过使用这两个函数,可以避免出现上述的ValueError错误。
需要注意的是,使用这些函数时需要将numpy数组作为参数传递给它们,而不能直接使用if语句或布尔运算符对数组进行判断。这样可以确保对多维数组的判断结果是准确的并避免出现歧义。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* [python-numpy-ValueError: The truth value of an array with more than one element is ambiguous.](https://blog.csdn.net/PSpiritV/article/details/124339801)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
- *3* [ValueError: The truth value of an array with more than one element is ambiguous. Use a.any() or a.al](https://blog.csdn.net/m0_57236802/article/details/128714704)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
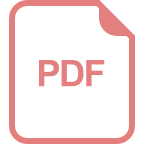
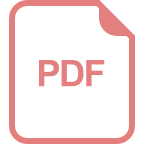
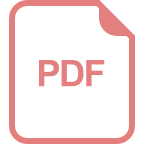



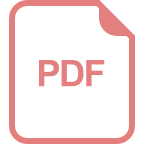
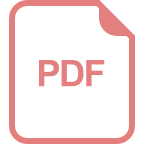
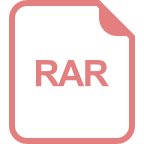
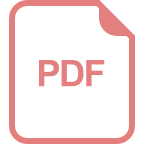
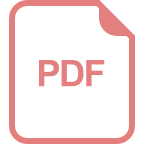
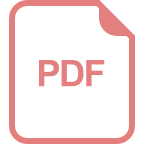
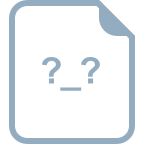