编写一个C++程序,数据加密程序,从键盘输入一个字符串(长度不大于20),输出其对应的密文。加密规则是:将字符串中每个字符的ASCII码值加100。再把加密的密文还原并输出来。
时间: 2024-12-16 10:13:59 浏览: 15
在C++中编写一个简单的数据加密程序,可以按照以下步骤操作:
```cpp
#include <iostream>
#include <string>
// 加密函数
std::string encrypt(const std::string& input) {
std::string encrypted;
for (char c : input) {
if (c >= 'a' && c <= 'z') { // ASCII码小写字母范围
encrypted += static_cast<char>((c + 100 - 'a' + 26) % 26 + 'a');
} else if (c >= 'A' && c <= 'Z') { // ASCII码大写字母范围
encrypted += static_cast<char>((c + 100 - 'A' + 26) % 26 + 'A');
} else { // 其他字符保持不变
encrypted += c;
}
}
return encrypted;
}
// 解密函数
std::string decrypt(const std::string& encrypted) {
std::string decrypted;
for (char c : encrypted) {
if (c >= 'a' && c <= 'z') { // 减去100并取模26
decrypted += static_cast<char>((c - 100 + 'a' - 26) % 26 + 'a');
} else if (c >= 'A' && c <= 'Z') {
decrypted += static_cast<char>((c - 100 + 'A' - 26) % 26 + 'A');
} else {
decrypted += c;
}
}
return decrypted;
}
int main() {
std::string message;
std::cout << "请输入一个不超过20个字符的字符串: ";
getline(std::cin, message); // 防止用户输入超出限制
if (message.length() > 20) {
std::cerr << "字符串过长,请输入不超过20个字符!\n";
return 1;
}
std::string encrypted = encrypt(message);
std::cout << "加密后的密文: " << encrypted << "\n";
std::string decrypted = decrypt(encrypted);
std::cout << "解密后的原文: " << decrypted << "\n";
return 0;
}
```
这个程序首先定义了两个辅助函数encrypt和decrypt,分别用于加密和解密。主函数中会提示用户输入字符串,然后加密并显示结果,最后解密并再次显示原始字符串。
阅读全文
相关推荐
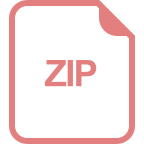
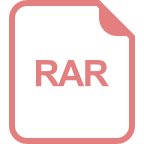
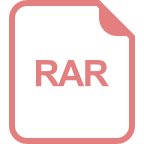
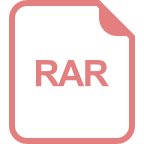
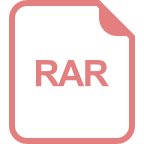
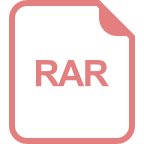
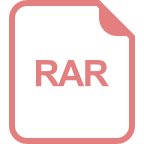
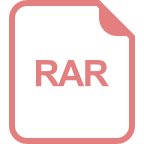
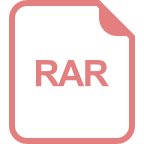
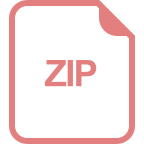
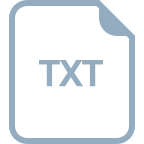
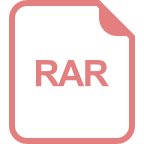
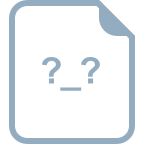
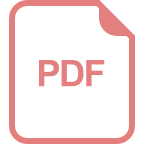
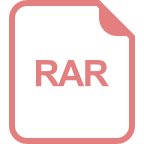
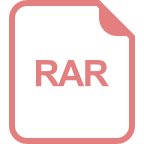