opencv识别圆头像
时间: 2023-08-31 13:08:32 浏览: 223
您好!要使用OpenCV识别圆形头像,您可以采用以下步骤:
1. 导入所需的库和模块:
```python
import cv2
import numpy as np
```
2. 读取图像并将其转换为灰度图像:
```python
image = cv2.imread('your_image.jpg')
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
```
3. 使用霍夫变换检测圆形:
```python
circles = cv2.HoughCircles(
gray, cv2.HOUGH_GRADIENT, dp=1, minDist=100, param1=50, param2=30, minRadius=10, maxRadius=50
)
```
4. 判断是否成功检测到圆形并进行后续处理:
```python
if circles is not None:
circles = np.uint16(np.around(circles))
for circle in circles[0, :]:
center = (circle[0], circle[1])
radius = circle[2]
# 在原始图像上绘制检测到的圆形
cv2.circle(image, center, radius, (0, 255, 0), 3)
else:
print("未检测到圆形头像!")
```
5. 显示结果:
```python
cv2.imshow('Circle Detection', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
请替换代码中的'your_image.jpg'为您要处理的图像路径。通过调整HoughCircles函数的参数可以对检测效果进行调优。希望对您有所帮助!如果您还有其他问题,请随时提问。
阅读全文
相关推荐

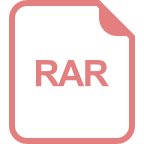
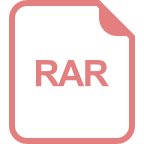
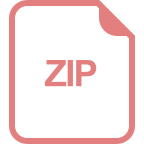
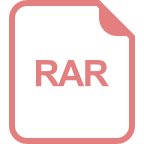
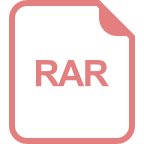
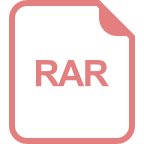
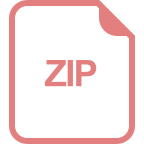
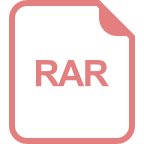
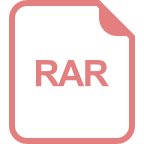
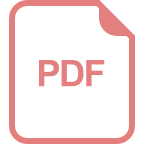
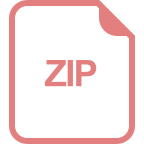
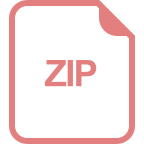
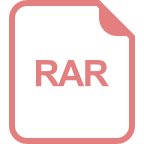
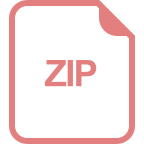
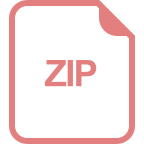