model.fit(x, y) ValueError: Expected 2D array, got 1D array instead:
时间: 2024-06-12 18:08:27 浏览: 157
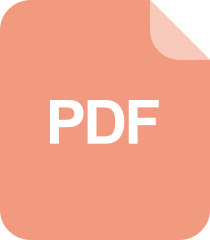
Python 3.x 新特性及10大变化
这个错误是由于您传递给 `model.fit` 方法的输入数据不符合预期格式所引起的。`model.fit` 方法期望输入一个二维数组,而您传递给它的是一个一维数组。
为了解决这个问题,您可以使用 `numpy` 的 `reshape` 方法将输入数组转换为二维形状。下面是一个示例代码:
```python
import numpy as np
x = np.array([1, 2, 3, 4, 5]) # 一维数组
y = np.array([0, 1, 0, 1, 0]) # 一维数组
x = x.reshape(-1, 1) # 将 x 转换为二维数组
model.fit(x, y) # 使用转换后的 x 进行训练
```
在这个示例中,我们使用 `reshape(-1, 1)` 将 `x` 的形状从 `(5,)` 转换为 `(5, 1)`,即一个列向量。
阅读全文
相关推荐
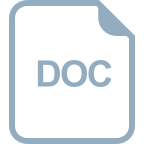
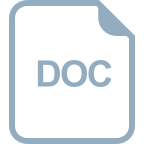

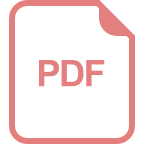










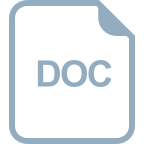
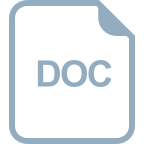
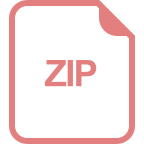