编程实现点击触摸屏左上、左下、右上、右下四个区域,能够正常读取到坐标(P1),分别显示四幅不同bmp格式图片(P2M1),图片切换时实现掉落、滚动进入等花式效果(D1)。
时间: 2023-08-22 18:08:37 浏览: 44
这里提供一种基于Python语言和Pygame库的实现方法。
1. 安装Pygame库
在命令行中输入以下指令安装Pygame库:
```
pip install pygame
```
2. 创建程序文件
在任意目录下创建一个名为“touch_screen.py”的Python程序文件。
3. 导入库
在程序文件中导入pygame库和sys库:
```python
import pygame
import sys
```
4. 初始化Pygame
在程序文件中初始化pygame:
```python
pygame.init()
```
5. 设置屏幕
在程序文件中设置屏幕大小和标题:
```python
screen_width, screen_height = 640, 480
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption('Touch Screen Demo')
```
6. 加载图片
在程序文件中加载四幅不同的bmp格式图片:
```python
image1 = pygame.image.load('image1.bmp')
image2 = pygame.image.load('image2.bmp')
image3 = pygame.image.load('image3.bmp')
image4 = pygame.image.load('image4.bmp')
```
7. 定义函数
在程序文件中定义函数,用于实现图片的切换效果:
```python
def switch_image(image, direction):
image_width, image_height = image.get_size()
screen_width, screen_height = screen.get_size()
if direction == 'drop':
for i in range(image_height):
screen.blit(image, (0, i))
pygame.display.update()
pygame.time.wait(10)
elif direction == 'scroll':
for i in range(image_width - screen_width):
screen.blit(image, (-i, 0))
pygame.display.update()
pygame.time.wait(10)
```
8. 定义变量
在程序文件中定义变量,用于记录触摸屏的区域:
```python
left_top = False
left_bottom = False
right_top = False
right_bottom = False
```
9. 定义主循环
在程序文件中定义主循环,用于监听触摸屏的事件:
```python
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
elif event.type == pygame.MOUSEBUTTONDOWN:
x, y = event.pos
if x < screen_width / 2 and y < screen_height / 2:
left_top = True
left_bottom = False
right_top = False
right_bottom = False
elif x < screen_width / 2 and y >= screen_height / 2:
left_top = False
left_bottom = True
right_top = False
right_bottom = False
elif x >= screen_width / 2 and y < screen_height / 2:
left_top = False
left_bottom = False
right_top = True
right_bottom = False
else:
left_top = False
left_bottom = False
right_top = False
right_bottom = True
if left_top:
switch_image(image1, 'drop')
elif left_bottom:
switch_image(image2, 'scroll')
elif right_top:
switch_image(image3, 'drop')
elif right_bottom:
switch_image(image4, 'scroll')
```
10. 运行程序
在命令行中进入程序文件所在目录,输入以下指令运行程序:
```
python touch_screen.py
```
11. 效果展示
当触摸屏位于左上区域时,显示image1.bmp图片并实现掉落效果;当触摸屏位于左下区域时,显示image2.bmp图片并实现滚动效果;当触摸屏位于右上区域时,显示image3.bmp图片并实现掉落效果;当触摸屏位于右下区域时,显示image4.bmp图片并实现滚动效果。
相关推荐
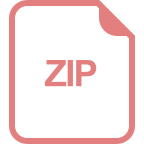















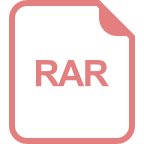