用js和MySQL数据库写一个在线题库系统
时间: 2024-06-09 11:07:04 浏览: 170
好的,下面是一个简单的在线题库系统的实现,使用了Node.js和MySQL数据库。
1. 安装Node.js和MySQL。
2. 创建一个项目文件夹,并在终端中进入该文件夹。
3. 初始化npm,创建一个package.json文件:
```
npm init
```
4. 安装依赖:
```
npm install express mysql ejs body-parser --save
```
5. 创建一个app.js文件,用于编写应用程序:
```javascript
const express = require('express');
const mysql = require('mysql');
const bodyParser = require('body-parser');
const ejs = require('ejs');
const app = express();
// 配置模板引擎
app.set('view engine', 'ejs');
app.set('views', __dirname + '/views');
// 解析请求体
app.use(bodyParser.urlencoded({ extended: false }));
// 连接MySQL数据库
const connection = mysql.createConnection({
host: 'localhost',
user: 'root',
password: '',
database: 'test'
});
connection.connect((error) => {
if (error) {
console.log('连接数据库失败:', error);
} else {
console.log('连接数据库成功');
}
});
// 首页路由
app.get('/', (req, res) => {
res.render('index');
});
// 添加题目路由
app.get('/add', (req, res) => {
res.render('add');
});
app.post('/add', (req, res) => {
const { title, options, answer } = req.body;
connection.query('INSERT INTO questions SET ?', { title, options, answer }, (error, results) => {
if (error) {
console.log('添加题目失败:', error);
res.send('添加题目失败');
} else {
console.log('添加题目成功:', results);
res.redirect('/');
}
});
});
// 题目列表路由
app.get('/list', (req, res) => {
connection.query('SELECT * FROM questions', (error, results) => {
if (error) {
console.log('查询题目列表失败:', error);
res.send('查询题目列表失败');
} else {
console.log('查询题目列表成功:', results);
res.render('list', { questions: results });
}
});
});
// 启动服务器
app.listen(3000, () => {
console.log('服务器已启动,监听端口3000');
});
```
6. 创建views文件夹,并在其中创建三个模板文件:index.ejs、add.ejs和list.ejs。
index.ejs:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>在线题库系统</title>
</head>
<body>
<h1>在线题库系统</h1>
<ul>
<li><a href="/add">添加题目</a></li>
<li><a href="/list">查看题目列表</a></li>
</ul>
</body>
</html>
```
add.ejs:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>添加题目</title>
</head>
<body>
<h1>添加题目</h1>
<form method="post" action="/add">
<p>
<label for="title">题目内容:</label>
<input type="text" id="title" name="title">
</p>
<p>
<label for="options">选项:</label>
<textarea id="options" name="options"></textarea>
</p>
<p>
<label for="answer">答案:</label>
<input type="text" id="answer" name="answer">
</p>
<button type="submit">提交</button>
</form>
</body>
</html>
```
list.ejs:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>题目列表</title>
</head>
<body>
<h1>题目列表</h1>
<table>
<thead>
<tr>
<th>题目ID</th>
<th>题目内容</th>
<th>选项</th>
<th>答案</th>
</tr>
</thead>
<tbody>
<% questions.forEach((question) => { %>
<tr>
<td><%= question.id %></td>
<td><%= question.title %></td>
<td><%= question.options %></td>
<td><%= question.answer %></td>
</tr>
<% }); %>
</tbody>
</table>
</body>
</html>
```
7. 创建一个MySQL数据库,创建一个questions表,用于存储题目数据:
```sql
CREATE TABLE questions (
id INT(11) NOT NULL AUTO_INCREMENT PRIMARY KEY,
title VARCHAR(255) NOT NULL,
options TEXT NOT NULL,
answer VARCHAR(255) NOT NULL
);
```
8. 运行应用程序:
```
node app.js
```
9. 在浏览器中访问http://localhost:3000,即可进入首页。从首页中可以进入添加题目页面和查看题目列表页面。
以上是一个简单的在线题库系统的实现,可以根据具体的需求进行修改和扩展。
阅读全文
相关推荐
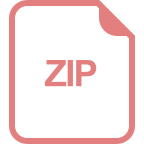
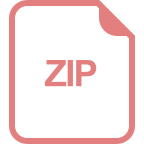

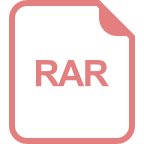
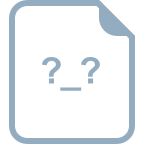
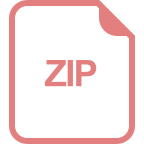
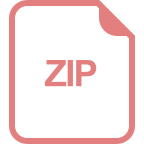
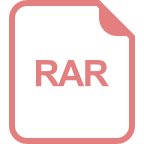
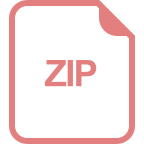
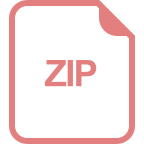
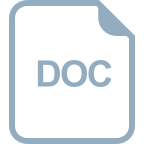
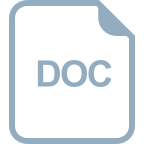
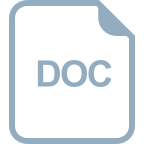
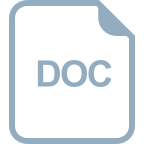
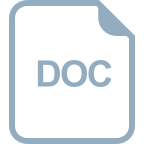
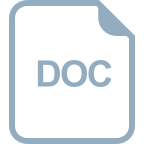
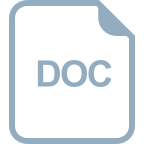
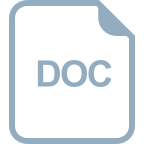
