在html5中使用内部js代码弹出对话框
时间: 2024-03-03 15:51:40 浏览: 40
可以使用以下代码在HTML5中使用内部JS代码弹出对话框:
```html
<!DOCTYPE html>
<html>
<head>
<title>弹出对话框</title>
</head>
<body>
<button onclick="alert('Hello World!')">点击我弹出对话框</button>
</body>
</html>
```
在上面的代码中,我们在按钮的onclick事件中调用了alert()方法来弹出对话框。当用户点击按钮时,将会弹出一个包含"Hello World!"文本的对话框。
相关问题
在HTML5页面使用内部js代码弹出对话框,内容为考生的姓名和学号
可以使用以下代码在HTML5页面中弹出对话框,其中包含考生的姓名和学号:
```html
<!DOCTYPE html>
<html>
<head>
<title>考生信息</title>
<script>
function showInfo() {
var name = "张三";
var id = "20210001";
alert("考生姓名:" + name + "\n学号:" + id);
}
</script>
</head>
<body>
<button onclick="showInfo()">点击查看考生信息</button>
</body>
</html>
```
在这个例子中,当用户点击按钮时,将调用JavaScript函数`showInfo()`,该函数将使用`alert()`函数弹出带有考生姓名和学号的对话框。在JavaScript函数中,我们定义了两个变量`name`和`id`,并将它们的值设置为考生的姓名和学号。在`alert()`函数中使用了字符串拼接符`+`和换行符`\n`来将考生姓名和学号组合在一起。
写一个点击按钮弹出对话框的前端代码
### 回答1:
```
<html>
<head>
<script>
function showDialog(){
alert("这是一个对话框");
}
</script>
</head>
<body>
<button onclick="showDialog()">点击按钮弹出对话框</button>
</body>
</html>
```
### 回答2:
以下是一个简单的前端代码示例,点击按钮后会弹出一个对话框:
```html
<!DOCTYPE html>
<html>
<head>
<title>点击按钮弹出对话框</title>
<script>
function showDialog() {
alert("这是一个对话框!");
}
</script>
</head>
<body>
<button onclick="showDialog()">点击这里</button>
</body>
</html>
```
这段代码首先定义了一个名为`showDialog()`的函数,函数内部使用`alert()`方法弹出一个对话框,显示一个简单的提示信息。
在HTML部分,创建了一个按钮,其中使用`onclick`属性指定了`showDialog()`函数,表示按钮被点击时调用该函数。因此,当用户点击按钮时,就会弹出一个对话框显示所指定的提示信息。
这只是一个简单的示例,实际情况中可能会使用更复杂的对话框组件,如模态框。但是基本原理是相同的,通过某种方式触发相应的事件,弹出对话框供用户交互。
### 回答3:
以下是一个简单的前端代码示例,点击按钮后弹出一个对话框。
```html
<!DOCTYPE html>
<html>
<head>
<title>点击按钮弹出对话框</title>
<style>
.dialog-container {
display: none;
position: fixed;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 300px;
height: 200px;
background-color: #fff;
border: 1px solid #ccc;
padding: 20px;
}
.dialog-title {
font-size: 18px;
font-weight: bold;
margin-bottom: 10px;
}
.dialog-buttons {
display: flex;
justify-content: flex-end;
}
.dialog-button {
margin-left: 10px;
}
</style>
</head>
<body>
<button id="open-dialog">点击弹出对话框</button>
<div class="dialog-container" id="dialog">
<div class="dialog-title">对话框标题</div>
<div class="dialog-content">对话框内容</div>
<div class="dialog-buttons">
<button class="dialog-button" id="dialog-confirm">确定</button>
<button class="dialog-button" id="dialog-cancel">取消</button>
</div>
</div>
<script>
const openDialogButton = document.querySelector('#open-dialog');
const dialog = document.querySelector('#dialog');
const dialogConfirmButton = document.querySelector('#dialog-confirm');
const dialogCancelButton = document.querySelector('#dialog-cancel');
openDialogButton.addEventListener('click', () => {
dialog.style.display = 'block';
});
dialogConfirmButton.addEventListener('click', () => {
dialog.style.display = 'none';
// 在确定按钮点击后执行其他逻辑
});
dialogCancelButton.addEventListener('click', () => {
dialog.style.display = 'none';
// 在取消按钮点击后执行其他逻辑
});
</script>
</body>
</html>
```
在这个示例中,我们创建了一个按钮和一个隐藏的对话框。当点击按钮时,通过JavaScript代码将对话框的display属性设置为block,使其显示出来。对话框的HTML结构包含一个标题、一个内容和两个按钮(确定和取消)。点击确定按钮将隐藏对话框并执行特定的逻辑,点击取消按钮仅隐藏对话框。您可以根据需要自定义对话框的样式和功能。
阅读全文
相关推荐
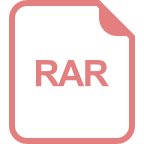
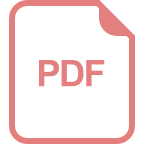
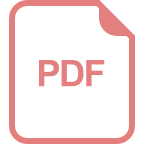
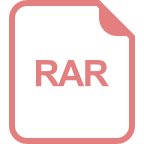
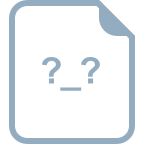
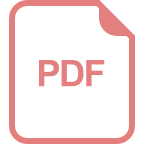
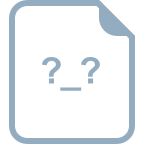
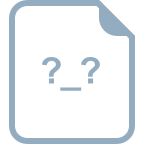
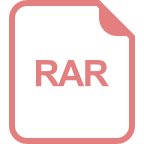
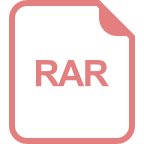
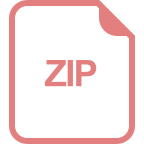
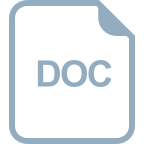
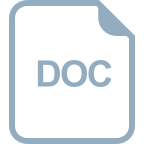