public class Machine { boolean isDanger; String name; public void setIsDanger(boolean boo) { isDanger = boo; } public boolean checkBag () //【代码0】声明异常 { DangerException danger=new DangerException() ; 【代码1】 //抛出danger }
时间: 2024-02-06 12:04:32 浏览: 106
在【代码0】处,可以声明一个 `throws DangerException` 来表示该方法可能会抛出一个 `DangerException` 异常。在【代码1】处,可以使用 `throw danger` 来抛出该异常。完整代码如下:
```
public class Machine {
boolean isDanger;
String name;
public void setIsDanger(boolean boo) {
isDanger = boo;
}
public boolean checkBag() throws DangerException {
DangerException danger = new DangerException();
throw danger;
}
}
```
相关问题
完成下列代码public class Goods { boolean isDanger; private String name; public void setIsDanger(boolean boo) { isDanger = boo; } public boolean isDanger() { return isDanger; } public void setName(String s) { name = s; } public String getName() { return name; }}DangerException.javapublic class DangerException extends Exception { String message; public DangerException() { message = "危险品!"; } public void toShow() { System.out.print(message+" "); }}Machine.javapublic class Machine { boolean isDanger; String name; public void setIsDanger(boolean boo) { isDanger = boo; } public boolean checkBag () //【代码0】声明异常 { DangerException danger=new DangerException() ; 【代码1】 //抛出danger }Check.javapublic class Check { public static void main(String args[]) { Machine machine = new Machine(); String name[] ={"苹果","炸药","西服","硫酸","手表","硫磺"}; Goods [] goods = new Goods[name.length]; //检查6件物品 for(int i= 0;i<name
.length;i++) { goods[i] = new Goods(); goods[i].setName(name[i]); if(i == 1 || i == 3 || i == 5) { goods[i].setIsDanger(true); machine.setIsDanger(true); } else { goods[i].setIsDanger(false); } try { boolean isDangerous = machine.checkBag(goods[i]); if(isDangerous) { System.out.println(goods[i].getName() + "是危险品!"); } else { System.out.println(goods[i].getName() + "是安全品!"); } } catch(DangerException e) { e.toShow(); System.out.println(goods[i].getName() + "是危险品!"); } } }}
其中【代码0】处需要声明DangerException异常,代码1处需要抛出DangerException异常。代码如下:
public boolean checkBag() throws DangerException {
if(isDanger) {
throw new DangerException();
}
else {
return false;
}
}
Check.java class Goods { boolean isDanger; String name; Goods(String name) { this.name = name; } public void setIsDanger(boolean boo) { isDanger = boo; } public boolean isDanger() { return isDanger; } public String getName() { return name; } } class DangerException extends Exception { String message; public DangerException() { message = "危险品!"; } public void toShow() { System.out.print(message+" "); } } class Machine { public void checkBag(Goods goods) throws DangerException { if(goods.isDanger()) { DangerException danger=new DangerException() ; 【代码1】 //抛出danger } } } public class Check { public static void main(String args[]) { Machine machine = new Machine(); Goods apple = new Goods("苹果"); apple.setIsDanger(false); Goods explosive = new Goods("炸药"); explosive.setIsDanger(true); try { machine.checkBag(explosive); System.out.println(explosive.getName()+"检查通过"); } catch(DangerException e) { 【代码2】 //e调用toShow()方法 System.out.println(explosive.getName()+"被禁止!"); } try { machine.checkBag(apple); System.out.println(apple.getName()+"检查通过"); } catch(DangerException e) { e.toShow(); System.out.println(apple.getName()+"被禁止!"); } } }
以下是您提供的Java代码,我来帮您补充缺失的部分:
```java
class Goods {
boolean isDanger;
String name;
Goods(String name) {
this.name = name;
}
public void setIsDanger(boolean boo) {
isDanger = boo;
}
public boolean isDanger() {
return isDanger;
}
public String getName() {
return name;
}
}
class DangerException extends Exception {
String message;
public DangerException() {
message = "危险品!";
}
public void toShow() {
System.out.print(message+" ");
}
}
class Machine {
public void checkBag(Goods goods) throws DangerException {
if(goods.isDanger()) {
DangerException danger = new DangerException();
throw danger; // 抛出danger
}
}
}
public class Check {
public static void main(String args[]) {
Machine machine = new Machine();
Goods apple = new Goods("苹果");
apple.setIsDanger(false);
Goods explosive = new Goods("炸药");
explosive.setIsDanger(true);
try {
machine.checkBag(explosive);
System.out.println(explosive.getName()+"检查通过");
} catch(DangerException e) {
e.toShow(); // e调用toShow()方法
System.out.println(explosive.getName()+"被禁止!");
}
try {
machine.checkBag(apple);
System.out.println(apple.getName()+"检查通过");
} catch(DangerException e) {
e.toShow();
System.out.println(apple.getName()+"被禁止!");
}
}
}
```
在上述代码中,我们定义了一个`Goods`类,其中包含一个`isDanger`属性表示该物品是否是危险品,以及一个`name`属性表示该物品的名称。`DangerException`类继承自`Exception`类,其中有一个`toShow()`方法用于输出“危险品!”的信息。`Machine`类中的`checkBag()`方法接收一个`Goods`对象作为参数,如果该对象是危险品,则抛出`DangerException`异常。
在主方法中,我们实例化了一个`Machine`对象和两个`Goods`对象,分别为苹果和炸药,并将炸药的`isDanger`属性设置为`true`,表示它是危险品。然后,我们在`try-catch`语句中调用`checkBag()`方法,如果抛出了`DangerException`异常,则在`catch`块中调用`toShow()`方法输出“危险品!”的信息,然后输出该物品的名称和“被禁止!”的信息。如果没有抛出异常,则输出该物品的名称和“检查通过”的信息。
阅读全文
相关推荐
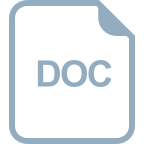
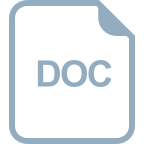
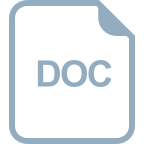
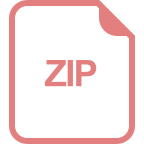
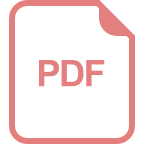











