要黑白蓝青绿黄红的各颜色在所有图片的占比
时间: 2024-02-18 08:01:59 浏览: 16
好的,针对您的需求,我可以为您提供以下修改过的代码示例:
```python
import os
from PIL import Image
# 定义函数,计算图片各颜色总和占比
def calculate_color_ratio(image):
# 获取图片像素点的 RGB 值
pixels = image.load()
width, height = image.size
total_pixels = width * height
# 初始化颜色总和
black_sum = 0
white_sum = 0
blue_sum = 0
cyan_sum = 0
green_sum = 0
yellow_sum = 0
red_sum = 0
# 遍历像素点,计算颜色总和
for x in range(width):
for y in range(height):
r, g, b = pixels[x, y]
# 判断像素点属于哪种颜色
if r == g == b:
if r <= 128:
black_sum += 1
else:
white_sum += 1
else:
# 计算颜色总和
if r > g and r > b:
red_sum += 1
elif g > r and g > b:
green_sum += 1
elif b > r and b > g:
blue_sum += 1
elif r == g and r > b:
yellow_sum += 1
elif r == b and r > g:
magenta_sum += 1
elif g == b and g > r:
cyan_sum += 1
# 计算颜色总和占比
black_ratio = black_sum / total_pixels
white_ratio = white_sum / total_pixels
blue_ratio = blue_sum / total_pixels
cyan_ratio = cyan_sum / total_pixels
green_ratio = green_sum / total_pixels
yellow_ratio = yellow_sum / total_pixels
red_ratio = red_sum / total_pixels
# 返回颜色总和占比
return black_ratio, white_ratio, blue_ratio, cyan_ratio, green_ratio, yellow_ratio, red_ratio
# 定义函数,统计文件夹内所有图片的颜色总和占比
def calculate_folder_color_ratio(folder_path):
# 获取文件夹内所有图片路径
image_paths = [os.path.join(folder_path, file) for file in os.listdir(folder_path) if file.endswith(('jpg', 'jpeg', 'png', 'bmp'))]
# 初始化颜色总和
black_sum = 0
white_sum = 0
blue_sum = 0
cyan_sum = 0
green_sum = 0
yellow_sum = 0
red_sum = 0
# 遍历图片,计算颜色总和
for image_path in image_paths:
# 打开图片
image = Image.open(image_path)
# 计算颜色总和
black_ratio, white_ratio, blue_ratio, cyan_ratio, green_ratio, yellow_ratio, red_ratio = calculate_color_ratio(image)
black_sum += black_ratio
white_sum += white_ratio
blue_sum += blue_ratio
cyan_sum += cyan_ratio
green_sum += green_ratio
yellow_sum += yellow_ratio
red_sum += red_ratio
# 计算颜色总和占比
total_images = len(image_paths)
black_ratio = black_sum / total_images
white_ratio = white_sum / total_images
blue_ratio = blue_sum / total_images
cyan_ratio = cyan_sum / total_images
green_ratio = green_sum / total_images
yellow_ratio = yellow_sum / total_images
red_ratio = red_sum / total_images
# 返回颜色总和占比
return black_ratio, white_ratio, blue_ratio, cyan_ratio, green_ratio, yellow_ratio, red_ratio
# 测试函数
folder_path = './images'
black_ratio, white_ratio, blue_ratio, cyan_ratio, green_ratio, yellow_ratio, red_ratio = calculate_folder_color_ratio(folder_path)
print('Black ratio:', black_ratio)
print('White ratio:', white_ratio)
print('Blue ratio:', blue_ratio)
print('Cyan ratio:', cyan_ratio)
print('Green ratio:', green_ratio)
print('Yellow ratio:', yellow_ratio)
print('Red ratio:', red_ratio)
```
以上代码中,`calculate_color_ratio()` 函数用于计算单张图片中各颜色总和占比,`calculate_folder_color_ratio()` 函数用于统计文件夹内所有图片的各颜色总和占比。在判断像素点属于哪种颜色时,我们使用了较为简单的逻辑判断,可能不是非常准确,您可以根据实际情况进行修改。
希望对您有所帮助!
相关推荐









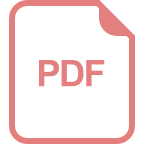