单链表的逆置c语言
时间: 2023-08-30 12:06:05 浏览: 94
单链表的逆置可以通过遍历链表,依次将每个节点的指针方向改为指向前一个节点来实现。以下是一个用C语言实现单链表逆置的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
struct Node* reverseList(struct Node* head) {
struct Node *prev = NULL, *current = head, *next = NULL;
while (current != NULL) {
next = current->next;
current->next = prev;
prev = current;
current = next;
}
return prev;
}
void printList(struct Node* head) {
while (head != NULL) {
printf("%d ", head->data);
head = head->next;
}
}
int main() {
struct Node *head = NULL, *newNode = NULL, *temp = NULL;
int n, i;
printf("Enter the number of nodes: ");
scanf("%d", &n);
// Create n nodes and link them together
for (i = 1; i <= n; i++) {
newNode = (struct Node*)malloc(sizeof(struct Node));
printf("Enter the data of node %d: ", i);
scanf("%d", &newNode->data);
newNode->next = NULL;
if (head == NULL) {
head = newNode;
}
else {
temp = head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
printf("Original list: ");
printList(head);
head = reverseList(head);
printf("\nReversed list: ");
printList(head);
return 0;
}
```
这段代码首先定义了一个 `Node` 结构体,包含了数据和下一个节点的指针。然后定义了一个 `reverseList` 函数,该函数接收一个单链表的头节点指针作为参数,并返回逆置后的头节点指针。函数中使用三个指针 `prev`、`current` 和 `next` 来遍历链表,依次将每个节点的指针方向改为指向前一个节点。最后返回 `prev` 指针,该指针指向逆置后的头节点。
在 `main` 函数中,首先读取用户输入的节点数,然后创建链表并打印原始链表。接着调用 `reverseList` 函数逆置链表,并打印逆置后的链表。
阅读全文
相关推荐
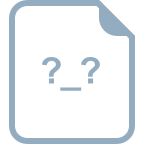








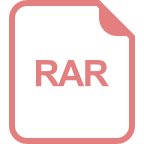
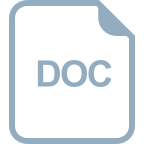




