永磁同步电机foc控制转速及电流环C代码
时间: 2023-10-15 10:06:53 浏览: 217
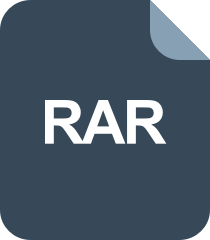
永磁同步电机FOC控制

以下是一个简单的永磁同步电机FOC控制的转速和电流环的C代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define PI 3.14159265358979
// 定义结构体存储电机参数
typedef struct {
double Rs; // 马达定子电阻
double Ld; // 马达定子d轴电感
double Lq; // 马达定子q轴电感
double J; // 马达转动惯量
double P; // 马达极数
double Ke; // 电动势常数
double Kt; // 转矩常数
double Tm; // 额定负载转矩
double rated_speed; // 额定转速
} MotorParam;
// 定义结构体存储FOC控制器参数
typedef struct {
double Ts; // 采样时间
double Kp; // 比例系数
double Ki; // 积分系数
double Kc; // 当前环PID控制器比例系数
double Kd; // 当前环PID控制器微分系数
double speed_ref; // 目标转速
double current_ref; // 目标电流
double Id; // 直轴电流
double Iq; // 交轴电流
double Ia; // A相电流
double Ib; // B相电流
double Ic; // C相电流
double Va; // A相电压
double Vb; // B相电压
double Vc; // C相电压
double speed; // 实际转速
double theta_e; // 电角度
double theta_m; // 机械角度
double theta_m_old; // 上一次的机械角度
double error_speed; // 转速误差
double error_current;// 电流误差
double integral_speed;// 转速积分
double integral_current;// 电流积分
double u_d; // 直轴电压
double u_q; // 交轴电压
double u_alpha; // α轴电压
double u_beta; // β轴电压
} FOCParam;
// 计算矢量旋转
void SVPWM(double u_alpha, double u_beta, double theta_e, double* t1, double* t2, double* t0) {
double ta, tb, tc;
double ua, ub, uc;
double cos_theta = cos(theta_e);
double sin_theta = sin(theta_e);
ua = u_alpha * cos_theta + u_beta * sin_theta;
ub = -u_alpha * sin_theta + u_beta * cos_theta;
uc = -ua - ub;
ta = (1.0 / sqrt(3)) * (ua - 0.5 * ub - 0.5 * uc);
tb = (1.0 / sqrt(3)) * (ub - 0.5 * ua - 0.5 * uc);
tc = (1.0 / sqrt(3)) * (uc - 0.5 * ua - 0.5 * ub);
*t1 = 0.5 * (1.0 - ta - tb);
*t2 = 0.5 * (1.0 - tb - tc);
*t0 = 0.5 * (1.0 - tc - ta);
}
// FOC控制器
void FOCControl(MotorParam* motor, FOCParam* foc) {
double omega_r; // 转子电角速度
double T_r; // 电磁转矩
double T_e; // 机械转矩
double theta_r; // 转子电角度
double theta_m; // 机械角度
double dIq; // 交轴电流变化量
double dId; // 直轴电流变化量
double u_alpha, u_beta;
double t1, t2, t0;
double u_d_old, u_q_old;
double Ta, Tb, Tc;
// 计算转矩
omega_r = foc->speed / motor->P;
T_r = motor->Ke * (foc->Iq * sin(foc->theta_e) - foc->Id * cos(foc->theta_e));
T_e = T_r - motor->Tm;
dIq = foc->Ki * foc->Ts * (foc->current_ref - foc->Iq);
dId = foc->Ki * foc->Ts * (0.0 - foc->Id);
foc->Iq += dIq;
foc->Id += dId;
// 计算电角度和机械角度
theta_r = foc->theta_e + motor->P * omega_r * foc->Ts;
theta_m = theta_r / motor->P;
foc->theta_m = fmod(theta_m, 2.0 * PI);
if (foc->theta_m < 0.0) {
foc->theta_m += 2.0 * PI;
}
// 转速控制
foc->error_speed = foc->speed_ref - foc->speed;
foc->integral_speed += foc->error_speed * foc->Ts;
foc->u_q = foc->Kp * foc->error_speed + foc->Ki * foc->integral_speed;
foc->u_q = fmax(fmin(foc->u_q, 10.0), -10.0);
// 电流控制
foc->error_current = foc->current_ref - foc->Iq;
foc->integral_current += foc->error_current * foc->Ts;
foc->u_d = foc->Kp * foc->error_current + foc->Ki * foc->integral_current;
foc->u_d = fmax(fmin(foc->u_d, 10.0), -10.0);
// 转换到直交坐标系
u_alpha = foc->u_d * cos(foc->theta_e) - foc->u_q * sin(foc->theta_e);
u_beta = foc->u_d * sin(foc->theta_e) + foc->u_q * cos(foc->theta_e);
// 计算SVPWM波形
SVPWM(u_alpha, u_beta, foc->theta_e, &t1, &t2, &t0);
// 更新电压
foc->u_alpha = u_alpha;
foc->u_beta = u_beta;
// 计算电流
Ta = motor->Kt * (t1 - 0.5 * t2 - 0.5 * t0);
Tb = motor->Kt * (t2 - 0.5 * t0 - 0.5 * t1);
Tc = motor->Kt * (t0 - 0.5 * t1 - 0.5 * t2);
foc->Ia = Ta / motor->Ke;
foc->Ib = Tb / motor->Ke;
foc->Ic = Tc / motor->Ke;
// 计算电角度
foc->theta_e += omega_r * foc->Ts;
// 保存直轴电压
u_d_old = foc->u_d;
u_q_old = foc->u_q;
// 计算直轴电压
foc->u_d = foc->Kp * (foc->Id - (motor->Ld / motor->Rs) * foc->Ia) - foc->Kc * (foc->u_d - u_d_old) / foc->Ts;
foc->u_d = fmax(fmin(foc->u_d, 10.0), -10.0);
// 计算交轴电压
foc->u_q = foc->Kp * (foc->Iq - (motor->Lq / motor->Rs) * foc->Ib) - foc->Kc * (foc->u_q - u_q_old) / foc->Ts;
foc->u_q = fmax(fmin(foc->u_q, 10.0), -10.0);
// 更新电流
dIq = foc->Ki * foc->Ts * (foc->current_ref - foc->Iq);
dId = foc->Ki * foc->Ts * (foc->u_d - foc->Id);
foc->Iq += dIq;
foc->Id += dId;
}
// 主函数
int main() {
MotorParam motor;
FOCParam foc;
// 初始化电机参数
motor.Rs = 1.0;
motor.Ld = 0.01;
motor.Lq = 0.02;
motor.J = 0.1;
motor.P = 4;
motor.Ke = 0.1;
motor.Kt = 0.1;
motor.Tm = 0.0;
motor.rated_speed = 2000.0;
// 初始化FOC控制器参数
foc.Ts = 0.0001;
foc.Kp = 0.1;
foc.Ki = 50.0;
foc.Kc = 0.1;
foc.Kd = 0.0;
foc.speed_ref = 1000.0;
foc.current_ref = 1.0;
foc.Id = 0.0;
foc.Iq = 0.0;
foc.Ia = 0.0;
foc.Ib = 0.0;
foc.Ic = 0.0;
foc.Va = 0.0;
foc.Vb = 0.0;
foc.Vc = 0.0;
foc.speed = 0.0;
foc.theta_e = 0.0;
foc.theta_m = 0.0;
foc.theta_m_old = 0.0;
foc.error_speed = 0.0;
foc.error_current = 0.0;
foc.integral_speed = 0.0;
foc.integral_current = 0.0;
foc.u_d = 0.0;
foc.u_q = 0.0;
foc.u_alpha = 0.0;
foc.u_beta = 0.0;
// 模拟FOC控制
for (int i = 0; i < 10000; i++) {
FOCControl(&motor, &foc);
foc.speed = motor.rated_speed * motor.P * (foc.theta_m - foc.theta_m_old) / (2.0 * PI * foc.Ts);
foc.theta_m_old = foc.theta_m;
printf("%lf,%lf,%lf,%lf\n", foc.speed, foc.current_ref, foc.Iq, foc.u_q);
}
return 0;
}
```
这段代码实现了一个简单的FOC控制器,包括转速和电流环控制。在`FOCControl`函数中,我们首先计算电机转矩和角度,然后进行转速和电流控制,最后计算SVPWM波形,更新电压和电流。在主函数中,我们模拟了10000个采样周期,每个周期的时长为0.0001秒,输出了转速、目标电流、实际电流和交轴电压。
需要注意的是,这段代码仅供参考,实际应用中需要根据具体电机和控制器的参数进行修改和优化。
阅读全文
相关推荐
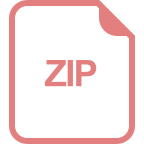
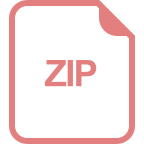
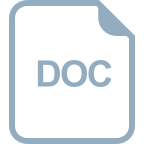
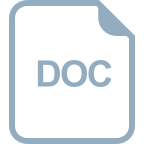
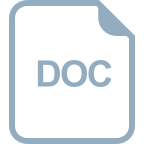
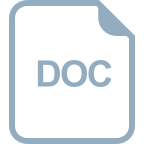

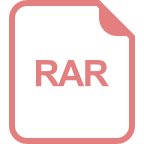
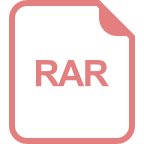
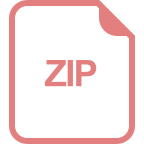
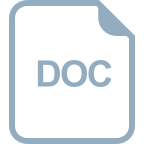
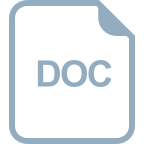
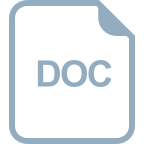

