vs2008上能使用fmemopen函数吗,如果不行,如何改写
时间: 2024-03-03 12:54:00 浏览: 188
在Visual Studio 2008中不支持fmemopen函数。该函数是一个POSIX标准函数,而Windows系统不直接支持POSIX标准。不过,你可以通过以下两种方式来替代fmemopen函数:
1. 使用tmpfile函数和fread/fwrite函数组合来实现类似的功能,具体实现如下:
```
#include <stdio.h>
#include <stdlib.h>
#define MEM_BUFFER_SIZE 1024
int main()
{
char* buffer = (char*)malloc(MEM_BUFFER_SIZE);
FILE* memFile = tmpfile();
fwrite(buffer, 1, MEM_BUFFER_SIZE, memFile);
// 将文件指针重新定位到文件开始位置
fseek(memFile, 0, SEEK_SET);
// 使用fread/fwrite函数操作内存缓冲区
fread(buffer, 1, MEM_BUFFER_SIZE, memFile);
fwrite(buffer, 1, MEM_BUFFER_SIZE, memFile);
fclose(memFile);
free(buffer);
return 0;
}
```
2. 使用WinAPI中的CreateFile函数和Memory-Mapped Files(内存映射文件)来实现类似的功能,具体实现如下:
```
#include <Windows.h>
#include <stdio.h>
#define MEM_BUFFER_SIZE 1024
int main()
{
HANDLE hFile = CreateFile(
"memfile.bin",
GENERIC_READ | GENERIC_WRITE,
0,
NULL,
CREATE_ALWAYS,
FILE_ATTRIBUTE_NORMAL,
NULL
);
HANDLE hMapping = CreateFileMapping(
hFile,
NULL,
PAGE_READWRITE,
0,
MEM_BUFFER_SIZE,
NULL
);
LPVOID lpBase = MapViewOfFile(
hMapping,
FILE_MAP_ALL_ACCESS,
0,
0,
MEM_BUFFER_SIZE
);
// 使用lpBase指针操作内存缓冲区
memcpy(lpBase, "hello, world!", 14);
printf("%s\n", (char*)lpBase);
UnmapViewOfFile(lpBase);
CloseHandle(hMapping);
CloseHandle(hFile);
return 0;
}
```
上面两种方式都可以实现在内存中操作文件的功能,你可以根据具体需求选择其中一种方式。
阅读全文
相关推荐
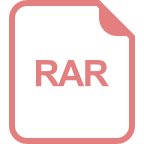
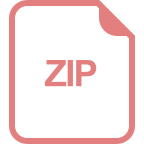
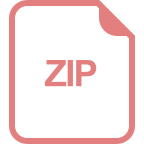
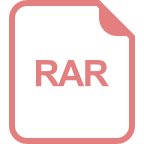
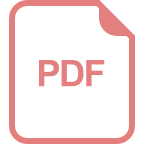
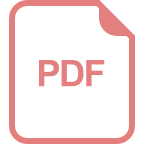
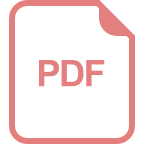
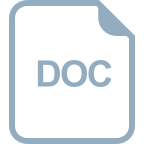
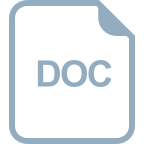
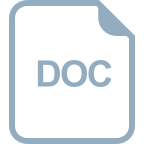
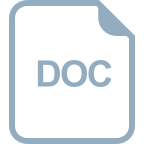
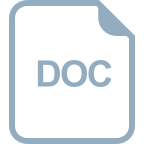
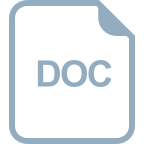
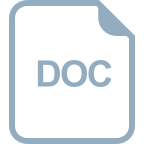
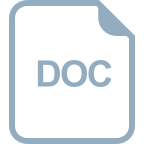
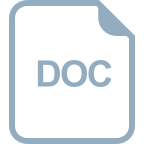
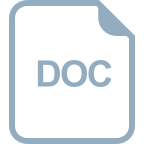