用数据结构设计图书管理系统
时间: 2024-03-13 21:39:30 浏览: 27
图书管理系统是一个常见的应用程序,它使用数据结构来组织和管理图书信息。下面是一个简单的示例,展示了如何使用数据结构设计一个图书管理系统:
1. 首先,我们可以使用结构体来定义图书的属性,例如书名、作者、出版社等。每本图书都可以表示为一个结构体对象。
```c
struct Book {
char title[100];
char author[100];
char publisher[100];
// 其他属性
};
```
2. 接下来,我们可以使用链表来存储图书的信息。每个节点都包含一个图书结构体对象和一个指向下一个节点的指针。
```c
struct Node {
struct Book book;
struct Node* next;
};
```
3. 然后,我们可以实现一些基本的操作,例如添加图书、删除图书和查找图书。这些操作可以通过遍历链表来完成。
```c
// 添加图书
void addBook(struct Node** head, struct Book book) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->book = book;
newNode->next = NULL;
if (*head == NULL) {
*head = newNode;
} else {
struct Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
// 删除图书
void deleteBook(struct Node** head, char* title) {
struct Node* current = *head;
struct Node* prev = NULL;
while (current != NULL) {
if (strcmp(current->book.title, title) == 0) {
if (prev == NULL) {
*head = current->next;
} else {
prev->next = current->next;
}
free(current);
return;
}
prev = current;
current = current->next;
}
}
// 查找图书
struct Book* findBook(struct Node* head, char* title) {
struct Node* current = head;
while (current != NULL) {
if (strcmp(current->book.title, title) == 0) {
return &(current->book);
}
current = current->next;
}
return NULL;
}
```
4. 最后,我们可以编写一个主函数来测试图书管理系统的功能。
```c
int main() {
struct Node* head = NULL;
// 添加图书
struct Book book1 = {"Book 1", "Author 1", "Publisher 1"};
addBook(&head, book1);
struct Book book2 = {"Book 2", "Author 2", "Publisher 2"};
addBook(&head, book2);
// 删除图书
deleteBook(&head, "Book 1");
// 查找图书
struct Book* foundBook = findBook(head, "Book 2");
if (foundBook != NULL) {
printf("Found book: %s\n", foundBook->title);
} else {
printf("Book not found\n");
}
return 0;
}
```
这是一个简单的图书管理系统的设计示例。你可以根据自己的需求和喜好进行扩展和修改。
相关推荐
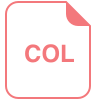
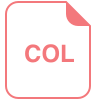
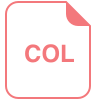
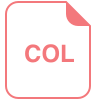
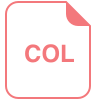









