c#与三菱plc通讯
时间: 2023-08-26 18:06:06 浏览: 209
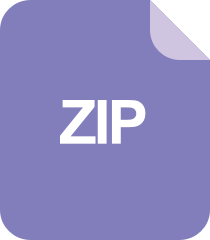
C#与三菱PLC串口通讯

你可以使用C#与三菱PLC进行通信。三菱PLC通信的常见方法包括使用串口通信和以太网通信。
对于串口通信,你可以使用C#的SerialPort类来实现与PLC的串口通信。首先,你需要确定PLC的串口参数(如波特率、数据位、停止位等),然后在C#中实例化一个SerialPort对象,设置好串口参数,然后打开串口进行通信。你可以使用SerialPort类的Read和Write方法来读取和写入数据。
对于以太网通信,你可以使用C#的Socket类来实现与PLC的以太网通信。首先,你需要确定PLC的IP地址和端口号,然后在C#中实例化一个Socket对象,设置好IP地址和端口号,然后连接到PLC。一旦连接成功,你可以使用Socket类的Send和Receive方法来发送和接收数据。
无论是串口通信还是以太网通信,你都需要了解PLC与C#之间的通信协议。三菱PLC通常使用Melsec协议进行通信。在C#中,你可以使用相关的库或者自己编写代码来实现Melsec协议的解析和封装。
总之,使用C#与三菱PLC进行通信需要了解PLC的通信参数和协议,并且熟悉C#中与串口和以太网通信相关的类和方法。
阅读全文
相关推荐
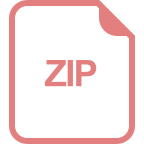
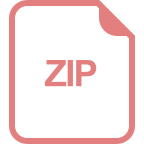
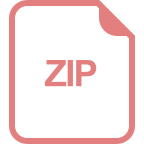
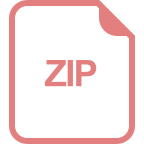
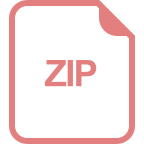
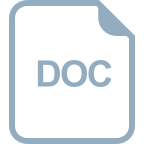
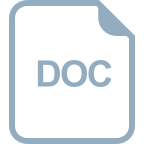
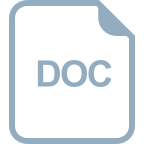

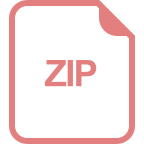
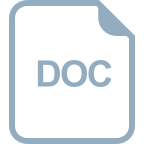
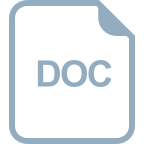
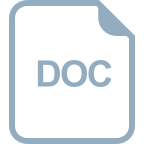