我现在有一块外部设备 ip地址192.168.10.67 写一段python代码,通过ssh的手段控制并进入设备
时间: 2024-03-22 22:42:50 浏览: 154
好的,以下是一段使用 Python 连接 SSH 并进入设备的示例代码:
```python
import paramiko
# 设置 SSH 连接参数
hostname = '192.168.10.67'
port = 22
username = '用户名'
password = '密码'
# 创建 SSH 客户端对象
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
# 连接设备
ssh.connect(hostname, port, username, password)
# 执行命令
stdin, stdout, stderr = ssh.exec_command('ls -l')
# 输出结果
print(stdout.read().decode())
# 关闭连接
ssh.close()
```
其中,`hostname` 为设备的 IP 地址,`port` 为 SSH 的端口号,默认为 22。`username` 和 `password` 分别为设备的登录名和密码。`paramiko` 是 Python 的 SSH 库,需要先安装才能使用。执行 `ls -l` 命令可以查看设备的文件列表,你可以将其替换为你需要执行的命令。
相关问题
import paramiko import pytest import psutil from common.abs_common import log @pytest.fixture(scope="module") def ssh_client(): print('-----ssh远程连接-------') ssh = paramiko.SSHClient() ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy()) ssh.connect('10.67.6.56', username='root', password='1') yield ssh ssh.close() print("获取PCIe设备的bus number,带宽和速度") def test_network_interface(ssh_client): stdin, stdout, stderr = ssh_client.exec_command("lspci|grep -i 'non' | cut -f1 -d ' '") interface = stdout.read().decode() stdin, stdout, stderr = ssh_client.exec_command(f"lspci -s {interface} -vvv |grep -i Lnkcap | cut -f2 -d ','") widthcap = stdout.read().decode().strip() outputwidthcap = stdout.read().decode("utf-8") stdin, stdout, stderr = ssh_client.exec_command(f"lspci -s {interface} -vvv |grep -i Lnksta | cut -f2 -d ','") widthsta = stdout.read().decode().strip() outputwidthsta = stdout.read().decode("utf-8") stdin, stdout, stderr = ssh_client.exec_command(f"lspci -s {interface} -vvv |grep -i Lnkcap | cut -f3 -d ','") speedcap = stdout.read().decode().strip() outputspeedcap = stdout.read().decode("utf-8") stdin, stdout, stderr = ssh_client.exec_command(f"lspci -s {interface} -vvv |grep -i Lnksta | cut -f3 -d ','") speedsta = stdout.read().decode().strip() outputspeedsta = stdout.read().decode("utf-8") print("比较lspci中PCIe设备的带宽和速度Lnkcap和Lnksta") assert widthcap == widthsta assert speedcap == speedsta print(outputwidthcap,outputwidthsta,outputspeedcap,outputspeedsta) if __name__ == '__main__': pytest.main(['-o log_cli=True --html=./test_get_nic_width.html -s'])
这段代码是用于测试获取PCIe设备的带宽和速度,其中使用了paramiko库建立远程SSH连接,执行了一些命令来获取相关信息。在test_network_interface函数中,首先使用lspci命令获取PCIe设备的bus number,然后通过grep和cut命令获取Lnkcap和Lnksta信息,分别表示PCIe设备的带宽和速度,最后通过assert语句比较这两个值是否相等。如果测试通过,会输出相关信息,并生成一个HTML格式的测试报告。
linux环境中将ssh -l gdplzy 10.67.12.51 -p 22 转换为可以执行登录的expect脚本
在 Linux 环境中,你可以使用 Expect 脚本来自动化 SSH 登录过程。这里是一个简单的例子,演示如何将提供的 SSH 命令转换成 Expect 脚本:
```bash
#!/usr/bin/expect
# 定义变量
username "gdplzy" # 用户名
hostname "10.67.12.51" # 主机地址
portnum "22" # 端口号
password "your_password_here" # 密码(你需要替换为你真实的密码)
# 启动 Expect 并连接到主机
spawn ssh -p $portnum
# 等待提示输入密码
expect "Password:"
# 发送密码
send "$password\r" # '\r' 表示回车键
# 检查登录是否成功,通常会显示欢迎信息
expect {
"\[.*\] Login correct" # 如果登录成功,期待这个模式
{ error_log_message "Login failed." }
}
# 登录成功后,可以添加更多的交互操作,或者在此处结束
# ...
# 最终关闭ssh连接
kill $expect_pid
```
在这个脚本里,`spawn` 命令启动了一个SSH会话,`expect` 和 `send` 分别用于等待密码提示并输入密码。注意,为了简化示例,我假设了密码不会被明文打印出来。在实际生产环境中,应该使用安全的方式来传递密码,例如环境变量或者加密后的文件。
阅读全文
相关推荐
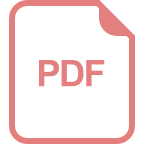
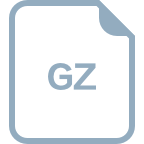
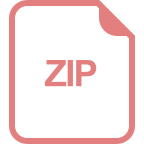
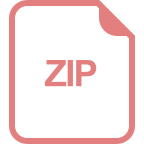
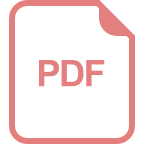
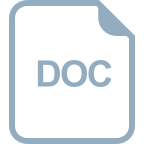
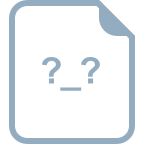
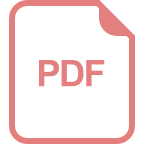
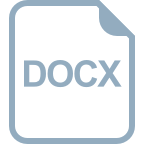
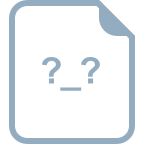
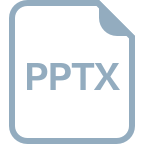
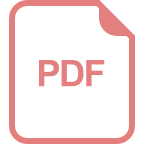
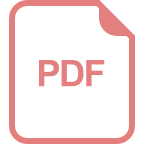