用Java编写一个应用程序,实现如下图所示的图形界面。要求选择左侧的选项后,右侧文字就呈现出对应的字体效果,且要求单击关闭按钮可将窗口关闭
时间: 2024-02-19 17:59:30 浏览: 101
好的,我可以帮你解决这个问题。
首先,你需要使用Java Swing库来创建图形用户界面。以下是一个简单的示例代码,用于创建一个窗口和关闭按钮:
```java
import javax.swing.*;
public class MyApplication {
public static void main(String[] args) {
JFrame frame = new JFrame("My Application");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JButton closeButton = new JButton("关闭");
closeButton.addActionListener(e -> frame.dispose());
frame.add(closeButton);
frame.pack();
frame.setVisible(true);
}
}
```
这个代码创建了一个带有一个“关闭”按钮的窗口。当单击该按钮时,窗口会被关闭。
接下来,你需要在窗口中添加一个下拉列表框和一个标签,用于显示所选字体的效果。以下是一个示例代码:
```java
import javax.swing.*;
import java.awt.*;
public class MyApplication {
public static void main(String[] args) {
JFrame frame = new JFrame("My Application");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 创建字体下拉列表框
JComboBox<String> fontComboBox = new JComboBox<>(new String[]{"Arial", "Verdana", "Times New Roman"});
fontComboBox.addActionListener(e -> {
// 更新标签的字体效果
Font font = new Font((String) fontComboBox.getSelectedItem(), Font.PLAIN, 24);
label.setFont(font);
label.setText("这是一段示例文本");
});
// 创建标签,用于显示字体效果
JLabel label = new JLabel("这是一段示例文本");
label.setFont(new Font("Arial", Font.PLAIN, 24));
// 创建关闭按钮
JButton closeButton = new JButton("关闭");
closeButton.addActionListener(e -> frame.dispose());
// 将控件添加到窗口中
JPanel panel = new JPanel();
panel.add(fontComboBox);
panel.add(label);
panel.add(closeButton);
frame.add(panel);
frame.pack();
frame.setVisible(true);
}
}
```
这个代码创建了一个带有一个下拉列表框、一个标签和一个“关闭”按钮的窗口。当选择下拉列表框中的选项时,标签会显示对应字体的效果。
你可以根据需要修改字体下拉列表框的选项,以及标签中显示的文本。同时,你也可以根据需要添加其他控件来扩展应用程序。
阅读全文
相关推荐
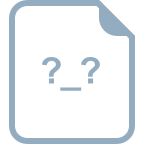

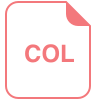
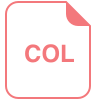
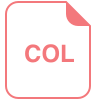
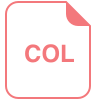
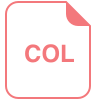
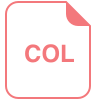
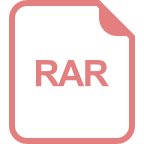
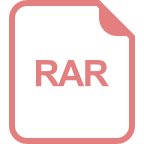
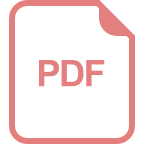
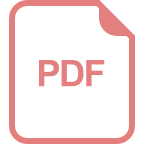
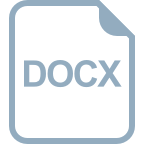
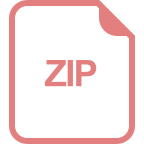
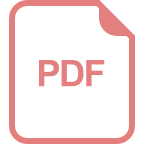