springboot中使用hutool工具包实现用户数据的导出导入功能,数据库使用mysql建表,代码详细实现过程
时间: 2024-05-12 19:18:37 浏览: 128
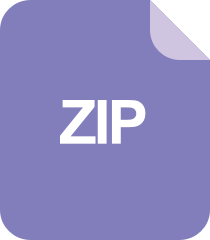
数据库设计库表结构导出工具+springboot构建+mysql库表导出
很好,我理解了你的问题。首先,我们需要添加hutool的依赖到pom.xml文件中,如下所示:
```
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>5.7.2</version>
</dependency>
```
然后,在Spring Boot项目中实现导入和导出功能的代码如下所示:
```
import cn.hutool.poi.excel.ExcelReader;
import cn.hutool.poi.excel.ExcelUtil;
import cn.hutool.poi.excel.ExcelWriter;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.multipart.MultipartFile;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.InputStream;
import java.net.URLEncoder;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@Controller
@RequestMapping("/user")
public class UserController {
/**
* 导出用户数据到Excel文件
*/
@GetMapping("/export")
public void export(HttpServletResponse response) throws IOException {
// 创建ExcelWriter对象
ExcelWriter writer = ExcelUtil.getWriter();
// 写入表头
writer.addHeaderAlias("id", "ID");
writer.addHeaderAlias("name", "姓名");
writer.addHeaderAlias("phone", "电话");
// 查询用户列表
List<User> userList = userService.list();
// 写入数据
writer.write(userList, true);
// 输出Excel文件
String fileName = "user.xlsx";
response.setContentType("application/vnd.ms-excel;charset=utf-8");
response.setHeader("Content-Disposition", "attachment;filename=" + URLEncoder.encode(fileName, "utf-8"));
writer.flush(response.getOutputStream());
writer.close();
}
/**
* 导入Excel文件中的用户数据
*/
@PostMapping("/import")
public Map<String, Object> imports(@RequestParam(value = "file") MultipartFile file) throws IOException {
// 创建ExcelReader对象
InputStream inputStream = file.getInputStream();
ExcelReader reader = ExcelUtil.getReader(inputStream);
// 读取Excel文件中的用户数据
List<User> userList = reader.readAll(User.class);
// 批量保存用户数据到数据库
userService.saveBatch(userList);
// 返回导入结果
Map<String, Object> result = new HashMap<>();
result.put("code", "success");
return result;
}
// UserService的声明和注入省略
}
```
在代码中,我们首先在UserController中定义了/export和/import两个接口,分别对应导出和导入功能。在导出接口中,我们使用ExcelWriter对象来生成Excel文件,并将用户列表写入到Excel文件中。在导入接口中,我们使用ExcelReader对象来读取上传的Excel文件,并将用户数据保存到数据库。
以上就是使用hutool工具包在Spring Boot中实现用户数据导入导出的全部内容。如果有任何问题,请告诉我。
阅读全文
相关推荐
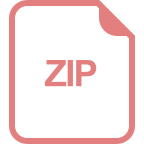
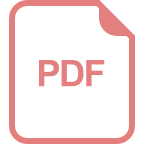
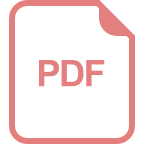
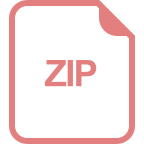
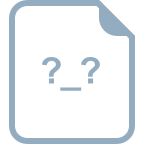
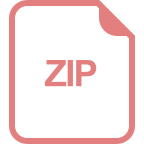
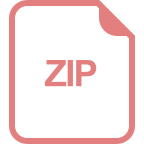
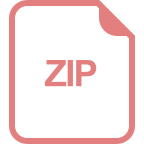
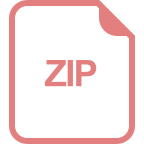
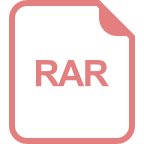
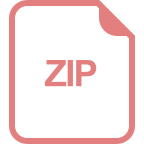
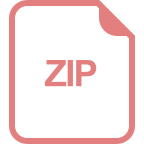
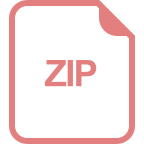
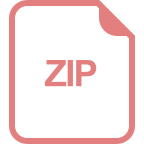
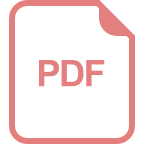
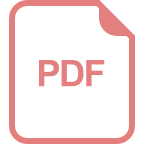
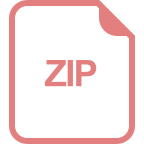
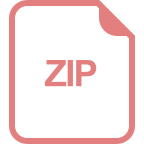