利用emwin图形库写一个x轴刻度为时间,y轴刻度为数据的实时曲线波形demo
时间: 2023-12-10 16:39:45 浏览: 31
这里提供一个基于emWin图形库的实时曲线波形demo,其中x轴刻度为时间,y轴刻度为数据。
首先,需要在开发板上安装emWin图形库,并且连接好显示器。接下来,我们需要编写一个C程序来实现实时曲线波形的绘制。
以下是示例代码:
```c
#include "GUI.h"
#include "DIALOG.h"
#define MAX_POINTS 100 // 最大数据点数
#define X_SIZE 240 // x轴长度
#define Y_SIZE 320 // y轴长度
#define X_OFFSET 40 // x轴偏移量
#define Y_OFFSET 40 // y轴偏移量
#define X_TICKS 5 // x轴刻度数
#define Y_TICKS 5 // y轴刻度数
// 数据点结构体
typedef struct {
int x;
int y;
} POINT;
// 数据点数组
static POINT _aPoint[MAX_POINTS];
// 数据点数
static int _NumPoints = 0;
// 绘制实时曲线波形
static void _DrawGraph(void) {
int i;
GUI_SetColor(GUI_RED);
GUI_DrawLine(X_OFFSET, Y_SIZE - Y_OFFSET, X_SIZE - X_OFFSET, Y_SIZE - Y_OFFSET);
GUI_DrawLine(X_OFFSET, Y_SIZE - Y_OFFSET, X_OFFSET, Y_OFFSET);
for (i = 0; i <= X_TICKS; i++) {
GUI_DrawLine(X_OFFSET + i * (X_SIZE - 2 * X_OFFSET) / X_TICKS,
Y_SIZE - Y_OFFSET, X_OFFSET + i * (X_SIZE - 2 * X_OFFSET) / X_TICKS,
Y_SIZE - Y_OFFSET + 4);
}
for (i = 0; i <= Y_TICKS; i++) {
GUI_DrawLine(X_OFFSET - 4, Y_SIZE - Y_OFFSET - i * (Y_SIZE - 2 * Y_OFFSET) / Y_TICKS,
X_OFFSET, Y_SIZE - Y_OFFSET - i * (Y_SIZE - 2 * Y_OFFSET) / Y_TICKS);
}
GUI_SetColor(GUI_GREEN);
for (i = 0; i < _NumPoints - 1; i++) {
GUI_DrawLine(_aPoint[i].x, _aPoint[i].y, _aPoint[i + 1].x, _aPoint[i + 1].y);
}
}
// 添加新的数据点
static void _AddPoint(int x, int y) {
if (_NumPoints >= MAX_POINTS) {
memmove(&_aPoint[0], &_aPoint[1], (MAX_POINTS - 1) * sizeof(POINT));
_NumPoints--;
}
_aPoint[_NumPoints].x = x;
_aPoint[_NumPoints].y = y;
_NumPoints++;
}
// 主函数
void MainTask(void) {
int i = 0;
GUI_Init();
while (1) {
// 模拟生成新的数据
int y = rand() % (Y_SIZE - 2 * Y_OFFSET) + Y_OFFSET;
_AddPoint(X_OFFSET + i * (X_SIZE - 2 * X_OFFSET) / X_TICKS, Y_SIZE - y);
i++;
if (i > X_TICKS) {
i = 0;
_NumPoints = 0;
}
// 绘制实时曲线波形
GUI_Clear();
_DrawGraph();
GUI_Delay(100);
}
}
```
上述代码中,我们定义了一个数据点结构体`POINT`,并且定义了一个数据点数组`_aPoint`,最大数据点数为100。`_NumPoints`变量表示当前数据点数。
`_DrawGraph`函数用于绘制实时曲线波形,其中通过GUI_DrawLine函数绘制出x轴和y轴,以及刻度线。`_AddPoint`函数用于添加新的数据点,并且如果数据点数超过最大值,则将最早的数据点删除。
在主函数中,我们模拟生成新的数据点,并且调用`_AddPoint`函数添加新的数据点。如果数据点数超过x轴刻度数,则将最早的数据点删除。最后,调用`_DrawGraph`函数绘制实时曲线波形。
通过上述代码,我们可以实现一个基于emWin图形库的实时曲线波形demo,其中x轴刻度为时间,y轴刻度为数据。
相关推荐
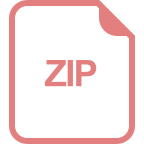
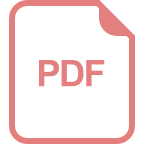














