c# dataGridView某一列的数据乘以2
时间: 2024-09-24 19:12:13 浏览: 45
在C#中,如果你想要将DataGridView控件中某一列的数据都乘以2,你可以通过以下几个步骤实现:
1. 首先,你需要获取到dataGridView的所有行数据。这通常需要遍历DataGridView的Rows集合。
```csharp
DataGridView dataGridView = // 你的DataGridView实例
foreach (DataGridViewRow row in dataGridView.Rows)
{
// 获取该行指定列的数据
object value = row.Cells["YourColumnHeader"].Value;
}
```
这里`"YourColumnHeader"`应该替换为你实际想操作的那一列的列名。
2. 然后,对获取到的数据执行乘以2的操作。如果值不是数字类型,可能会引发异常,因此通常会添加一些条件检查。
```csharp
if (value is double || value is decimal) // 或者其他数值类型
{
double multipliedValue = Convert.ToDouble(value) * 2;
row.Cells["YourColumnHeader"].Value = multipliedValue;
}
else
{
Console.WriteLine($"无法处理非数值类型的数据: {value}");
}
```
3. 如果你想一次性处理所有列,可以考虑使用Linq或者 LINQ to Objects 来简化操作:
```csharp
// 使用Linq
var modifiedRows = dataGridView.Rows.Cast<DataGridViewRow>()
.Where(row => row.Cells["YourColumnHeader"].Value != null)
.Select(row => {
var value = Convert.ToDouble(row.Cells["YourColumnHeader"].Value);
return new DataGridViewCell() { Value = value * 2 };
}).ToList();
// 将修改后的单元格应用回对应行
foreach (DataGridViewCell cell in modifiedRows)
{
row.Cells(cell.ColumnIndex).Value = cell.Value;
}
```
记得在实际项目中替换 `"YourColumnHeader"` 为你需要操作的实际列头名,并确保代码在运行时有正确的权限访问和修改DataGridView。
阅读全文
相关推荐







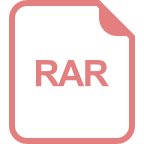
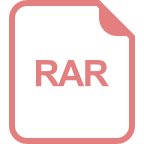





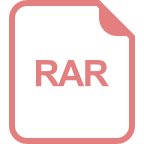



