#print(texts1) texts2=[[word[0] for word in text if word[1]=='n' or word[1]=='v' or word[1]=='a' or word[1]=='d' or word[1]=='nr' or word[1]=='nrfg' or word[1]=='ns' or word[1]=='nt' or word[1]=='nz' or word[1]=='vn' or word[1]=='vd' or word[1]=='an' or word[1]=='ad' or word[1]=='l'] for text in texts1]
时间: 2023-10-11 13:09:38 浏览: 27
这段代码是对上一个问题中的分词结果texts1进行了过滤,只保留了名词(n)、动词(v)、形容词(a)、副词(d)、人名(nr)、人名(音译)(nrfg)、地名(ns)、机构名(nt)、其他专名(nz)、动名词(vn)、趋向动词(vd)、形容词性语素(an)、副形词性语素(ad)和习用语(l)这些词性的词语,将结果以列表形式存储到texts2中。
具体来说,代码中使用了列表推导式,先遍历texts1中的每个text,再遍历text中的每个word,使用if条件语句对word的词性进行判断,如果满足要求则将word的原始词语加入到列表中,最终得到的是一个二维列表,其中每个子列表对应原来的一个文本,包含了所有满足条件的词语。
相关问题
print(texts2) texts3 =[[word for word in text if len(word)>1]for text in texts2] print(texts3)
这段代码是对上一步得到的符合条件的词语进行过滤,只保留长度大于1的词语,并将结果存储在一个二维列表texts3中。其中,每个子列表对应一个文本,其中包含了该文本中符合条件且长度大于1的词语。
这是一种常见的文本预处理方法,通过对文本进行分词、词性标注和过滤等操作,可以得到一组干净、规范的词语,为后续的文本分析和机器学习任务提供了基础数据。
print(texts2) texts3 =[[word for word in text if len(word)>1]for text in texts2] print(texts3) texts5 = [' '.join(text)for text in texts3] df['textcut'] = texts5 print(df) file = [] for word in texts5: file.extend(word) print(file)
这段代码是将上一步得到的texts2中的词语进行了进一步过滤,并将结果保存到DataFrame中。
具体来说,代码中首先使用列表推导式对texts2中的每个子列表进行进一步过滤,只保留长度大于1的词语,将结果存储到texts3中,并使用了列表推导式将texts3中的每个子列表中的词语连接成一个字符串,用空格分隔开,存储到texts5中。
然后,通过DataFrame的操作,将texts5添加到DataFrame中的新列textcut中。
最后,使用for循环遍历texts5中的每个字符串,将其中的词语逐一添加到file列表中,得到一个包含所有词语的列表。
综上,这段代码的作用是将一组文本进行了分词、词性过滤、长度过滤和字符串拼接等处理,得到了一个包含所有词语的列表file,可以用于后续的文本分析。
相关推荐
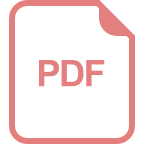
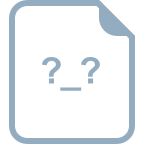
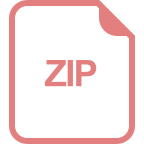












