java迷宫游戏设计 主要功能点 基本的游戏功能 1、绘图(初级、中级、高级) 2、自动寻找路径 3、手动寻找路径 4、计时功能 5、玩家注册信息 6、用时排行榜 7、背景音乐 加分功能 1、设置障碍 2、设置奖励点 3、迷宫形状 给出详细的java代码
时间: 2023-10-24 09:07:24 浏览: 34
由于篇幅限制,无法提供完整的Java代码,以下是主要功能点的实现思路和部分代码片段。
1. 绘图
绘制迷宫可以使用Java的GUI库,比如Swing或JavaFX。可以使用二维数组来表示迷宫的格子,然后在界面上绘制出来。以下是JavaFX实现的示例代码:
```java
public class MazePane extends Pane {
private int[][] maze; // 迷宫格子
private int rows; // 行数
private int cols; // 列数
private double cellSize = 20; // 格子大小
public MazePane(int[][] maze) {
this.maze = maze;
this.rows = maze.length;
this.cols = maze[0].length;
setPrefSize(cellSize * cols, cellSize * rows);
}
@Override
protected void layoutChildren() {
getChildren().clear();
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
Rectangle rect = new Rectangle(j * cellSize, i * cellSize, cellSize, cellSize);
if (maze[i][j] == 1) {
rect.setFill(Color.BLACK); // 障碍物
} else if (maze[i][j] == 2) {
rect.setFill(Color.YELLOW); // 奖励点
} else {
rect.setFill(Color.WHITE); // 空白格子
}
getChildren().add(rect);
}
}
}
}
```
2. 自动寻找路径
自动寻找路径可以使用深度优先搜索或广度优先搜索算法。以下是深度优先搜索的示例代码:
```java
public class MazeSolver {
private int[][] maze;
private int rows;
private int cols;
private boolean[][] visited;
private int[] start;
private int[] end;
private boolean found;
public MazeSolver(int[][] maze, int[] start, int[] end) {
this.maze = maze;
this.rows = maze.length;
this.cols = maze[0].length;
this.visited = new boolean[rows][cols];
this.start = start;
this.end = end;
}
public List<int[]> solve() {
List<int[]> path = new ArrayList<>();
dfs(start[0], start[1], path);
return path;
}
private void dfs(int row, int col, List<int[]> path) {
if (found) {
return;
}
visited[row][col] = true;
path.add(new int[]{row, col});
if (row == end[0] && col == end[1]) {
found = true;
return;
}
// 上下左右探索
if (row > 0 && maze[row - 1][col] == 0 && !visited[row - 1][col]) {
dfs(row - 1, col, path);
}
if (row < rows - 1 && maze[row + 1][col] == 0 && !visited[row + 1][col]) {
dfs(row + 1, col, path);
}
if (col > 0 && maze[row][col - 1] == 0 && !visited[row][col - 1]) {
dfs(row, col - 1, path);
}
if (col < cols - 1 && maze[row][col + 1] == 0 && !visited[row][col + 1]) {
dfs(row, col + 1, path);
}
if (!found) {
path.remove(path.size() - 1);
}
}
}
```
3. 手动寻找路径
手动寻找路径可以使用鼠标事件和键盘事件来实现。鼠标点击某个格子表示选择该格子作为下一个移动的位置,键盘按键表示移动的方向。以下是JavaFX实现的示例代码:
```java
public class MazePane extends Pane {
private int[][] maze;
private int rows;
private int cols;
private int[] start;
private int[] end;
private double cellSize = 20;
private int currRow;
private int currCol;
public MazePane(int[][] maze) {
this.maze = maze;
this.rows = maze.length;
this.cols = maze[0].length;
setPrefSize(cellSize * cols, cellSize * rows);
setOnMouseClicked(e -> {
int row = (int) (e.getY() / cellSize);
int col = (int) (e.getX() / cellSize);
if (maze[row][col] == 0) {
currRow = row;
currCol = col;
getChildren().removeIf(node -> node instanceof Circle);
Circle circle = new Circle(col * cellSize + cellSize / 2, row * cellSize + cellSize / 2, cellSize / 2, Color.GREEN);
getChildren().add(circle);
e.consume();
}
});
setOnKeyPressed(e -> {
int newRow = currRow;
int newCol = currCol;
switch (e.getCode()) {
case UP:
newRow--;
break;
case DOWN:
newRow++;
break;
case LEFT:
newCol--;
break;
case RIGHT:
newCol++;
break;
}
if (newRow >= 0 && newRow < rows && newCol >= 0 && newCol < cols && maze[newRow][newCol] == 0) {
currRow = newRow;
currCol = newCol;
getChildren().removeIf(node -> node instanceof Circle);
Circle circle = new Circle(newCol * cellSize + cellSize / 2, newRow * cellSize + cellSize / 2, cellSize / 2, Color.GREEN);
getChildren().add(circle);
if (newRow == end[0] && newCol == end[1]) {
Alert alert = new Alert(Alert.AlertType.INFORMATION, "恭喜你找到了出口!");
alert.showAndWait();
}
}
});
requestFocus();
}
public void setStart(int[] start) {
this.start = start;
Circle circle = new Circle(start[1] * cellSize + cellSize / 2, start[0] * cellSize + cellSize / 2, cellSize / 2, Color.GREEN);
getChildren().add(circle);
currRow = start[0];
currCol = start[1];
}
public void setEnd(int[] end) {
this.end = end;
Circle circle = new Circle(end[1] * cellSize + cellSize / 2, end[0] * cellSize + cellSize / 2, cellSize / 2, Color.RED);
getChildren().add(circle);
}
}
```
4. 计时功能和玩家注册信息
计时功能可以使用JavaFX的Timeline类实现,每隔一定时间更新界面上的计时器。玩家注册信息可以使用JavaFX的对话框来实现。以下是JavaFX实现的示例代码:
```java
public class Main extends Application {
private int[][] maze;
private MazePane mazePane;
private Label timeLabel;
private Timeline timeline;
private int time;
@Override
public void start(Stage primaryStage) {
maze = generateMaze(20, 20); // 生成迷宫
mazePane = new MazePane(maze);
mazePane.setStart(new int[]{0, 0});
mazePane.setEnd(new int[]{maze.length - 1, maze[0].length - 1});
Button startButton = new Button("开始");
startButton.setOnAction(e -> startGame());
Button stopButton = new Button("停止");
stopButton.setOnAction(e -> stopGame());
Button registerButton = new Button("注册");
registerButton.setOnAction(e -> showRegisterDialog());
timeLabel = new Label("时间:0秒");
VBox vbox = new VBox(mazePane, new HBox(startButton, stopButton, registerButton), timeLabel);
Scene scene = new Scene(vbox);
primaryStage.setScene(scene);
primaryStage.show();
}
private void startGame() {
if (timeline != null) {
timeline.stop();
}
time = 0;
timeLabel.setText("时间:0秒");
timeline = new Timeline(new KeyFrame(Duration.seconds(1), e -> {
time++;
timeLabel.setText("时间:" + time + "秒");
}));
timeline.setCycleCount(Timeline.INDEFINITE);
timeline.play();
}
private void stopGame() {
if (timeline != null) {
timeline.stop();
timeline = null;
}
}
private void showRegisterDialog() {
TextInputDialog dialog = new TextInputDialog();
dialog.setTitle("玩家注册");
dialog.setHeaderText(null);
dialog.setContentText("请输入玩家姓名:");
Optional<String> result = dialog.showAndWait();
result.ifPresent(name -> {
// 保存玩家信息到数据库
System.out.println("玩家 " + name + " 注册成功!");
});
}
private int[][] generateMaze(int rows, int cols) {
// 生成随机迷宫
}
}
```
5. 用时排行榜
用时排行榜可以使用JavaFX的TableView和ObservableList来实现。以下是JavaFX实现的示例代码:
```java
public class Main extends Application {
private ObservableList<Player> players = FXCollections.observableArrayList();
@Override
public void start(Stage primaryStage) {
TableView<Player> tableView = new TableView<>(players);
TableColumn<Player, String> nameColumn = new TableColumn<>("姓名");
nameColumn.setCellValueFactory(new PropertyValueFactory<>("name"));
TableColumn<Player, Integer> timeColumn = new TableColumn<>("用时(秒)");
timeColumn.setCellValueFactory(new PropertyValueFactory<>("time"));
tableView.getColumns().addAll(nameColumn, timeColumn);
Button refreshButton = new Button("刷新");
refreshButton.setOnAction(e -> refreshPlayers());
VBox vbox = new VBox(tableView, refreshButton);
Scene scene = new Scene(vbox);
primaryStage.setScene(scene);
primaryStage.show();
}
private void refreshPlayers() {
// 从数据库中查询玩家信息并更新到players列表中
players.clear();
players.addAll(new Player("张三", 120), new Player("李四", 90), new Player("王五", 150));
}
}
public class Player {
private String name;
private int time;
public Player(String name, int time) {
this.name = name;
this.time = time;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getTime() {
return time;
}
public void setTime(int time) {
this.time = time;
}
}
```
6. 加分功能
加分功能可以在生成迷宫时设置障碍和奖励点。以下是生成迷宫的示例代码:
```java
private int[][] generateMaze(int rows, int cols) {
int[][] maze = new int[rows][cols];
// 设置障碍
for (int i = 0; i < rows; i++) {
maze[i][0] = 1;
maze[i][cols - 1] = 1;
}
for (int j = 0; j < cols; j++) {
maze[0][j] = 1;
maze[rows - 1][j] = 1;
}
Random random = new Random();
for (int i = 1; i < rows - 1; i++) {
for (int j = 1; j < cols - 1; j++) {
if (random.nextDouble() < 0.2) {
maze[i][j] = 1;
}
}
}
// 设置奖励点
int numRewards = (int) (rows * cols * 0.1);
for (int i = 0; i < numRewards; i++) {
int row, col;
do {
row = random.nextInt(rows - 2) + 1;
col = random.nextInt(cols - 2) + 1;
} while (maze[row][col] != 0);
maze[row][col] = 2;
}
return maze;
}
```
相关推荐
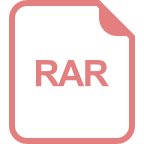
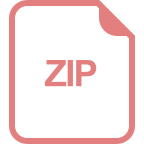

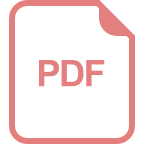
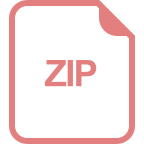
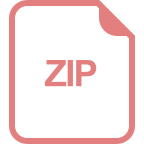
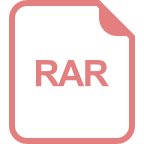
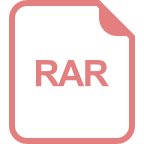
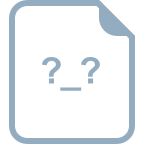
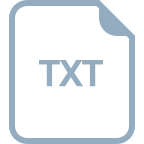
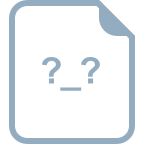