netty无法远程连接
时间: 2024-02-21 14:24:45 浏览: 22
如果Netty无法进行远程连接,可能存在以下几种原因:
1. 网络连接问题:请确保你的网络连接正常,可以使用ping命令测试你要连接的主机是否可以访问。
2. 防火墙问题:如果你的网络中存在防火墙,请确保防火墙允许你的Netty应用程序进行网络连接。你可以尝试关闭防火墙或者在防火墙中添加规则来允许Netty应用程序进行网络连接。
3. 代码问题:请检查你的代码是否正确。例如,你是否正确地设置了Bootstrap或ServerBootstrap,是否正确地设置了ChannelInitializer,是否正确地处理了入站和出站的消息等等。
如果你检查了以上几点,仍然无法进行远程连接,可以尝试在代码中添加一些日志或者调试信息,以便更好地了解问题出在哪里。
相关问题
netty如何在远程连接
Netty可以通过使用SocketChannel实现远程连接。在客户端上,你可以使用Bootstrap创建一个SocketChannel,然后连接到服务端。例如,下面的代码片段展示了如何连接到一个远程的TCP服务端:
```java
EventLoopGroup group = new NioEventLoopGroup();
try {
Bootstrap b = new Bootstrap();
b.group(group)
.channel(NioSocketChannel.class)
.handler(new MyClientInitializer());
ChannelFuture f = b.connect("remotehost", port).sync();
f.channel().closeFuture().sync();
} finally {
group.shutdownGracefully();
}
```
在服务端上,你可以使用ServerBootstrap创建一个ServerSocketChannel,然后等待客户端连接。例如,下面的代码片段展示了如何启动一个TCP服务端:
```java
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new MyServerInitializer());
ChannelFuture f = b.bind(port).sync();
f.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
```
在上面的代码中,MyClientInitializer和MyServerInitializer是用来初始化ChannelPipeline的类。这些类包含了一些ChannelHandler,用来处理入站和出站的消息。你可以根据你的需要添加更多的ChannelHandler来实现你的业务逻辑。
netty如何才能进行远程连接
Netty可以通过使用SocketChannel实现远程连接。在客户端上,你可以使用Bootstrap创建一个SocketChannel,然后连接到服务端。例如,下面的代码片段展示了如何连接到一个远程的TCP服务端:
```java
EventLoopGroup group = new NioEventLoopGroup();
try {
Bootstrap b = new Bootstrap();
b.group(group)
.channel(NioSocketChannel.class)
.handler(new MyClientInitializer());
ChannelFuture f = b.connect("remotehost", port).sync();
f.channel().closeFuture().sync();
} finally {
group.shutdownGracefully();
}
```
在服务端上,你可以使用ServerBootstrap创建一个ServerSocketChannel,然后等待客户端连接。例如,下面的代码片段展示了如何启动一个TCP服务端:
```java
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new MyServerInitializer());
ChannelFuture f = b.bind(port).sync();
f.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
```
在上面的代码中,MyClientInitializer和MyServerInitializer是用来初始化ChannelPipeline的类。这些类包含了一些ChannelHandler,用来处理入站和出站的消息。你可以根据你的需要添加更多的ChannelHandler来实现你的业务逻辑。
相关推荐
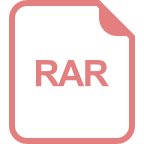
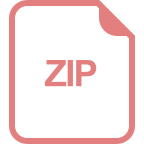
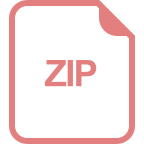
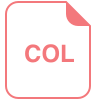
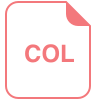
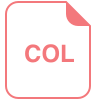









