编写一个servlet,在servlet中查询goods表中的记录,并在showMoveGoods.jsp页面中逆序显示偶数行的记录。在本例中需要使用实体类goods,但需要为Goods添加一个属性rowno封装行号。
时间: 2024-01-22 14:21:12 浏览: 20
首先,我们需要创建一个Goods类,并在其中添加一个rowno属性。
```java
public class Goods {
private int id;
private String name;
private double price;
private int rowno;
// getter and setter methods
}
```
接下来,我们需要编写一个servlet来查询goods表中的记录,并将结果传递给showMoveGoods.jsp页面。在servlet中,我们可以使用JDBC来从数据库中检索数据,并将结果存储在Goods对象列表中。然后,我们可以为每个Goods对象设置rowno属性,并将结果传递给showMoveGoods.jsp页面。
```java
@WebServlet("/showMoveGoods")
public class ShowMoveGoodsServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
try {
// get a connection to the database
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "root", "password");
// create a statement
Statement stmt = conn.createStatement();
// execute the query
ResultSet rs = stmt.executeQuery("SELECT * FROM goods");
// create a list to store the goods
List<Goods> goodsList = new ArrayList<>();
// iterate through the result set and create a Goods object for each row
int rowno = 1;
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
double price = rs.getDouble("price");
// create a Goods object
Goods goods = new Goods();
goods.setId(id);
goods.setName(name);
goods.setPrice(price);
goods.setRowno(rowno++);
// add the Goods object to the list
goodsList.add(goods);
}
// close the result set, statement, and connection
rs.close();
stmt.close();
conn.close();
// set the goods list as an attribute of the request
request.setAttribute("goodsList", goodsList);
// forward the request to the showMoveGoods.jsp page
RequestDispatcher dispatcher = request.getRequestDispatcher("showMoveGoods.jsp");
dispatcher.forward(request, response);
} catch (SQLException ex) {
ex.printStackTrace();
}
}
}
```
在showMoveGoods.jsp页面中,我们可以使用JSTL标签库来逆序显示偶数行的记录。我们可以使用<c:forEach>标签遍历Goods对象列表,并使用<c:if>标签检查当前行是否为偶数行。如果是偶数行,则显示该行的数据。
```jsp
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<!DOCTYPE html>
<html>
<head>
<title>Show Move Goods</title>
</head>
<body>
<h1>Show Move Goods</h1>
<table border="1">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Price</th>
</tr>
</thead>
<tbody>
<c:forEach items="${goodsList}" var="goods" varStatus="status">
<c:if test="${status.index % 2 == 1}">
<tr>
<td>${goods.rowno}</td>
<td>${goods.name}</td>
<td>${goods.price}</td>
</tr>
</c:if>
</c:forEach>
</tbody>
</table>
</body>
</html>
```
当用户访问/showMoveGoods时,servlet将查询goods表中的记录,并将结果传递给showMoveGoods.jsp页面。在页面中,只有偶数行的数据将被逆序显示。
相关推荐
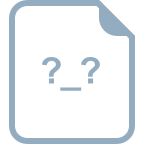












