输入n个整数,求其中最大的3个数和。c++
时间: 2024-01-27 18:05:53 浏览: 47
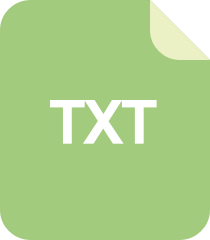
求3个整数中的最大值
可以使用堆来解决这个问题,具体的做法是:
1. 建立一个大小为3的小根堆(因为我们要找的是最大的3个数,所以要用小根堆)。
2. 依次读入n个整数,如果堆的大小小于3,直接将当前数插入堆中;否则,如果当前数比堆顶元素大,则弹出堆顶元素,将当前数插入堆中。
3. 最后将堆中的元素相加即为所求的最大的3个数和。
以下是C++代码实现:
```cpp
#include <iostream>
#include <queue>
using namespace std;
int main() {
int n;
cin >> n;
priority_queue<int, vector<int>, greater<int>> q; //小根堆
while (n--) {
int x;
cin >> x;
if (q.size() < 3) {
q.push(x);
} else {
if (x > q.top()) {
q.pop();
q.push(x);
}
}
}
int sum = 0;
while (!q.empty()) {
sum += q.top();
q.pop();
}
cout << sum << endl;
return 0;
}
```
时间复杂度为 O(nlog3),空间复杂度为 O(3)。
阅读全文
相关推荐
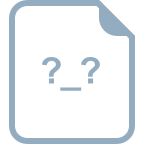
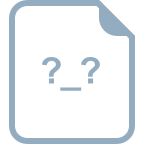
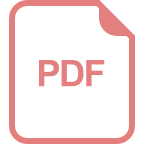
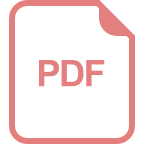
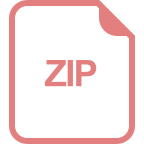
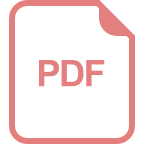
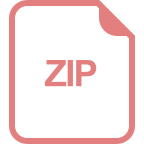
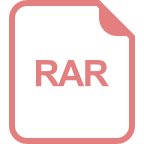
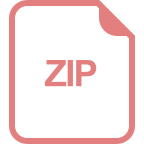
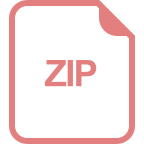