qt 点击按钮绘制矩形ROI
时间: 2024-09-05 10:02:26 浏览: 109
在Qt中实现点击按钮绘制矩形ROI(Region of Interest)通常涉及到信号与槽机制以及绘图相关的类。ROI可以用于图像处理中选择特定区域进行分析或操作。以下是实现该功能的基本步骤:
1. **创建Qt Widgets Application**:首先,使用Qt Creator创建一个新的Widgets Application项目。
2. **设计界面**:在主窗口中添加一个`QGraphicsView`控件,用于展示图像和ROI。添加一个按钮用于触发ROI的绘制。
3. **设置信号与槽**:将按钮的点击信号连接到一个槽函数,这个槽函数将负责启动ROI的绘制。
4. **实现ROI绘制**:在槽函数中,可以使用`QGraphicsScene`和`QGraphicsView`来添加和管理ROI。通过重写`mousePressEvent`事件处理函数来监听鼠标点击事件,然后在适当的坐标位置绘制矩形ROI。
5. **设置ROI属性**:可以对ROI进行调整,比如改变颜色、透明度等,以适应不同的应用场景。
下面是一个简化的代码示例:
```cpp
// MyWidget.h
#ifndef MYWIDGET_H
#define MYWIDGET_H
#include <QWidget>
#include <QPushButton>
#include <QGraphicsScene>
#include <QGraphicsView>
class MyWidget : public QWidget {
Q_OBJECT
public:
MyWidget(QWidget *parent = nullptr);
~MyWidget();
private slots:
void onDrawRectClicked();
private:
QPushButton *drawRectButton;
QGraphicsScene *scene;
QGraphicsView *view;
};
#endif // MYWIDGET_H
// MyWidget.cpp
#include "MyWidget.h"
#include <QVBoxLayout>
#include <QGraphicsRectItem>
MyWidget::MyWidget(QWidget *parent) : QWidget(parent) {
drawRectButton = new QPushButton("Draw Rectangle", this);
scene = new QGraphicsScene(this);
view = new QGraphicsView(scene, this);
QVBoxLayout *layout = new QVBoxLayout(this);
layout->addWidget(drawRectButton);
layout->addWidget(view);
connect(drawRectButton, &QPushButton::clicked, this, &MyWidget::onDrawRectClicked);
}
MyWidget::~MyWidget() {
}
void MyWidget::onDrawRectClicked() {
// Assuming the user clicked the button to draw the rectangle,
// this slot function will be called. Here, we're not handling
// the actual mouse events for drawing the rectangle, which
// would require further handling in `mousePressEvent`, etc.
// Create a rectangle item
QGraphicsRectItem *rectItem = scene->addRect(0, 0, 50, 50);
// Set the rectangle properties, such as color, pen style, etc.
QPen pen(Qt::red);
pen.setWidth(2);
rectItem->setPen(pen);
}
```
在这个示例中,当用户点击"Draw Rectangle"按钮时,会调用`onDrawRectClicked`槽函数,在`QGraphicsScene`中添加一个矩形。实际的矩形绘制还需要进一步处理鼠标事件来确定矩形的位置和大小。
阅读全文
相关推荐
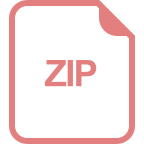
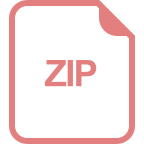
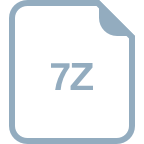
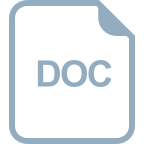


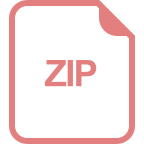
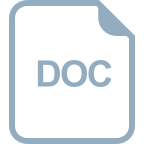
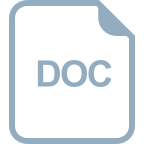
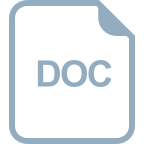



