python如何设置qt5文本框回车键跳到一个文本框并全选文本框的内容
时间: 2023-06-09 20:07:32 浏览: 73
可以使用Qt的signals和slots机制来实现回车键跳转文本框并全选其中的内容。具体实现代码可以参考以下示例:
```python
from PyQt5.QtCore import Qt, pyqtSignal
from PyQt5.QtGui import QKeyEvent
from PyQt5.QtWidgets import QApplication, QLineEdit, QVBoxLayout, QWidget
class MyLineEdit(QLineEdit):
enter_pressed = pyqtSignal()
def __init__(self, parent=None):
super().__init__(parent)
def keyPressEvent(self, event: QKeyEvent):
if event.key() == Qt.Key_Return or event.key() == Qt.Key_Enter:
self.enter_pressed.emit()
return
super().keyPressEvent(event)
class MyWidget(QWidget):
def __init__(self, parent=None):
super().__init__(parent)
self.lineedit1 = MyLineEdit(self)
self.lineedit1.enter_pressed.connect(self.focus_lineedit2)
self.lineedit2 = MyLineEdit(self)
self.lineedit2.enter_pressed.connect(self.focus_lineedit1)
layout = QVBoxLayout(self)
layout.addWidget(self.lineedit1)
layout.addWidget(self.lineedit2)
def focus_lineedit2(self):
self.lineedit1.clearFocus()
self.lineedit2.selectAll()
self.lineedit2.setFocus()
def focus_lineedit1(self):
self.lineedit2.clearFocus()
self.lineedit1.selectAll()
self.lineedit1.setFocus()
if __name__ == '__main__':
import sys
app = QApplication(sys.argv)
widget = MyWidget()
widget.show()
sys.exit(app.exec_())
```
以上代码创建了两个MyLineEdit对象,并将它们放在MyWidget上。对于每一个MyLineEdit对象,我们定义了一个名为enter_pressed的signal,当按下回车键时触发。在MyLineEdit的keyPressEvent()函数中,我们判断当按下回车键时,触发enter_pressed signal。在MyWidget中,我们实现了两个focus_lineedit函数,分别用于设置焦点在第一个LineEdit和第二个LineEdit上。在这两个函数中,我们使用selectAll()函数全选文本框的内容,使用clearFocus()函数清除其他文本框上的焦点,再使用setFocus()函数设置焦点在当前文本框上。
最后,运行以上代码可以看到,当按下第一个LineEdit上的回车键,焦点会自动跳转到第二个LineEdit上,并且第二个LineEdit中的文本会被全选。按下第二个LineEdit上的回车键,焦点又会跳转回第一个LineEdit上,并且第一个LineEdit中的文本会被全选。
相关推荐
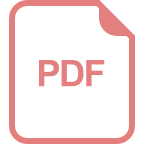
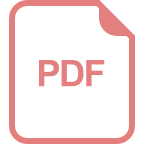














