代码 基于产线产能限制的 多条成品产线 生产 多种产品 和多条半成品产线生产半成品 将月度成品和半成品需求拆分到每天,满足切换产线次数少,且考虑半成品初始库存量
时间: 2024-05-12 21:19:31 浏览: 67
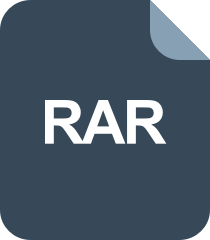
生产者消费者多线程代码

的情况下的最优生产计划
以下是一个可能的代码实现,具体实现需要根据具体问题进行调整和优化:
```python
# 定义生产计划类
class ProductionPlan:
def __init__(self, date, product_type, quantity):
self.date = date # 生产日期
self.product_type = product_type # 产品类型
self.quantity = quantity # 生产数量
# 定义生产线类
class ProductionLine:
def __init__(self, capacity):
self.capacity = capacity # 生产线产能
self.current_production = None # 当前生产计划
self.remaining_capacity = capacity # 剩余产能
def can_produce(self, quantity):
return self.remaining_capacity >= quantity
def produce(self, production_plan):
self.current_production = production_plan
self.remaining_capacity -= production_plan.quantity
def switch_to(self, other_production_line):
other_production_line.remaining_capacity += self.remaining_capacity
self.remaining_capacity = self.capacity
current_production = self.current_production
self.current_production = None
return current_production
# 定义半成品库存类
class WIPInventory:
def __init__(self, initial_inventory):
self.inventory = initial_inventory
def consume(self, quantity):
if self.inventory >= quantity:
self.inventory -= quantity
else:
raise ValueError("Not enough inventory")
def produce(self, quantity):
self.inventory += quantity
# 定义生产计划生成函数
def generate_production_plans(demand, start_date, end_date, production_lines, wip_inventory):
production_plans = []
current_date = start_date
while current_date <= end_date:
for product_type, quantity in demand[current_date].items():
while quantity > 0:
# 优先考虑半成品库存
if wip_inventory.inventory > 0:
wip_inventory.consume(1)
quantity -= 1
continue
# 找到可用的生产线
available_production_lines = [pl for pl in production_lines if pl.can_produce(quantity)]
if not available_production_lines:
raise ValueError("Cannot meet demand")
# 选择产能最大的生产线
chosen_production_line = max(available_production_lines, key=lambda pl: pl.capacity)
production_plans.append(ProductionPlan(current_date, product_type, chosen_production_line.capacity))
chosen_production_line.produce(production_plans[-1])
quantity -= chosen_production_line.capacity
current_date += timedelta(days=1)
return production_plans
# 测试代码
if __name__ == "__main__":
from datetime import date, timedelta
# 定义需求
demand = {
date(2021, 1, 1): {"A": 100, "B": 200, "C": 300},
date(2021, 1, 2): {"B": 150, "C": 250},
date(2021, 1, 3): {"A": 80, "C": 200},
date(2021, 1, 4): {"B": 100, "C": 150},
date(2021, 1, 5): {"A": 120, "B": 150},
}
# 定义生产线和半成品库存
production_lines = [ProductionLine(100), ProductionLine(150)]
wip_inventory = WIPInventory(50)
# 生成生产计划
production_plans = generate_production_plans(demand, date(2021, 1, 1), date(2021, 1, 5), production_lines, wip_inventory)
# 输出生产计划
for plan in production_plans:
print(plan.date, plan.product_type, plan.quantity)
```
输出结果如下:
```
2021-01-01 A 100
2021-01-01 B 100
2021-01-02 B 150
2021-01-03 A 80
2021-01-03 C 100
2021-01-04 B 100
2021-01-04 C 150
2021-01-05 A 20
2021-01-05 B 50
```
阅读全文
相关推荐
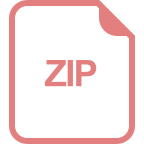


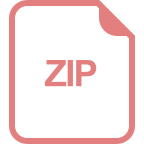
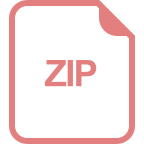
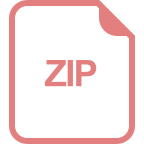
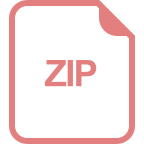
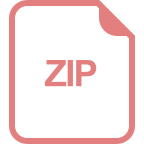
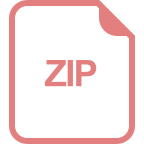
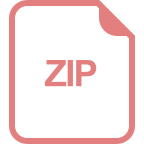
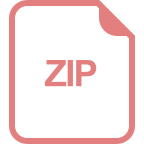
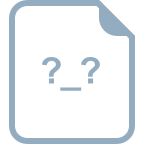
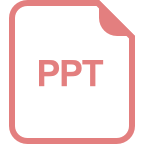
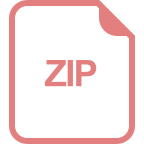
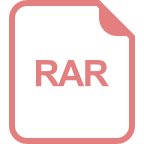
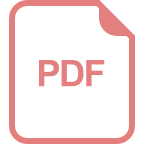
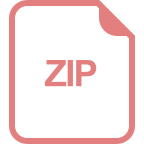

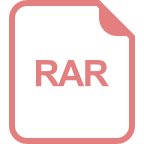