定时任务中有多线程怎么暂停任务还停止线程
时间: 2023-07-04 12:31:02 浏览: 156
如果一个定时任务中有多个线程在执行,你需要在任务暂停时,停止所有线程的执行,可以通过以下步骤实现:
1. 在定时任务中维护一个 boolean 类型的变量 isRunning,用于标识任务是否正在运行。
2. 在任务启动时,将 isRunning 设置为 true,并在任务执行的主循环中检查该变量的值,如果为 false,则退出循环。
3. 在任务暂停时,将 isRunning 设置为 false,并等待所有线程执行完毕。
4. 在等待线程执行完毕的过程中,需要定时检查 isRunning 的值,如果为 true,则立即返回。
5. 在所有线程执行完毕后,可以停止定时任务的执行。
以下是示例代码:
```java
public class MyJob implements Job {
private volatile boolean isRunning;
public void execute(JobExecutionContext context) throws JobExecutionException {
isRunning = true;
// 启动线程
Thread thread1 = new Thread(new MyRunnable());
Thread thread2 = new Thread(new MyRunnable());
thread1.start();
thread2.start();
// 任务主循环
while (isRunning) {
// do something
// 如果 isRunning 为 false,则跳出循环
}
// 等待线程执行完毕
while (thread1.isAlive() || thread2.isAlive()) {
if (!isRunning) {
// 如果 isRunning 为 false,则立即返回
return;
}
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
// ignore
}
}
// 停止定时任务的执行
JobExecutionException e = new JobExecutionException("job stopped");
e.setUnscheduleAllTriggers(true);
throw e;
}
public void pause() {
isRunning = false;
}
}
class MyRunnable implements Runnable {
public void run() {
while (true) {
// do something
if (Thread.interrupted()) {
// 如果收到中断信号,则停止执行
break;
}
}
}
}
```
在上面的示例代码中,MyJob 类实现了 Quartz 的 Job 接口,并在其中启动了两个线程。在任务暂停时,将 isRunning 设置为 false,并等待所有线程执行完毕。线程在执行过程中,定时检查中断信号,如果收到中断信号,则停止执行。在所有线程执行完毕后,通过抛出 JobExecutionException 异常停止定时任务的执行。
阅读全文
相关推荐
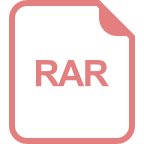
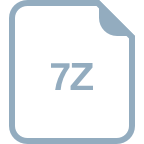
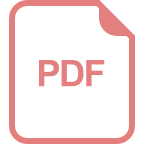
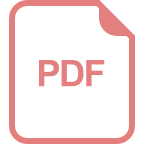
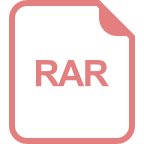
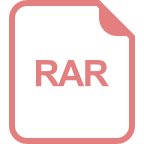
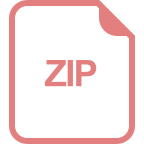
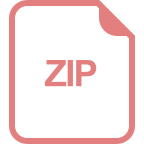
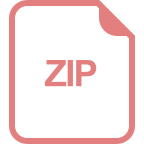
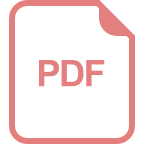
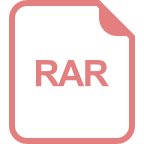
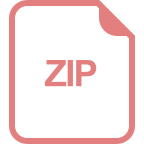
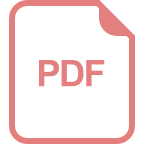
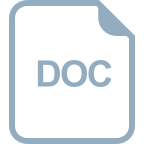
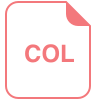

