Java创建一个学生类 包括studentId, studentName, studentGender, studentBirth, studentPhone, studentAddress
时间: 2023-10-10 12:07:58 浏览: 184
下面是一个Java创建学生类的示例代码:
```java
public class Student {
private int studentId;
private String studentName;
private String studentGender;
private String studentBirth;
private String studentPhone;
private String studentAddress;
public Student(int studentId, String studentName, String studentGender, String studentBirth, String studentPhone, String studentAddress) {
this.studentId = studentId;
this.studentName = studentName;
this.studentGender = studentGender;
this.studentBirth = studentBirth;
this.studentPhone = studentPhone;
this.studentAddress = studentAddress;
}
// Getter and Setter methods
public int getStudentId() {
return studentId;
}
public void setStudentId(int studentId) {
this.studentId = studentId;
}
public String getStudentName() {
return studentName;
}
public void setStudentName(String studentName) {
this.studentName = studentName;
}
public String getStudentGender() {
return studentGender;
}
public void setStudentGender(String studentGender) {
this.studentGender = studentGender;
}
public String getStudentBirth() {
return studentBirth;
}
public void setStudentBirth(String studentBirth) {
this.studentBirth = studentBirth;
}
public String getStudentPhone() {
return studentPhone;
}
public void setStudentPhone(String studentPhone) {
this.studentPhone = studentPhone;
}
public String getStudentAddress() {
return studentAddress;
}
public void setStudentAddress(String studentAddress) {
this.studentAddress = studentAddress;
}
}
```
在上述代码中,我们定义了一个名为 `Student` 的类,包括了 `studentId`、`studentName`、`studentGender`、`studentBirth`、`studentPhone` 和 `studentAddress` 这6个属性。我们使用了构造方法来初始化这些属性,并提供了相应的 Getter 和 Setter 方法来获取和设置这些属性的值。
使用示例代码如下:
```java
public class Main {
public static void main(String[] args) {
Student student = new Student(1, "Tom", "male", "2000-01-01", "1234567890", "123 Main St.");
System.out.println("Student ID: " + student.getStudentId());
System.out.println("Student Name: " + student.getStudentName());
System.out.println("Student Gender: " + student.getStudentGender());
System.out.println("Student Birth: " + student.getStudentBirth());
System.out.println("Student Phone: " + student.getStudentPhone());
System.out.println("Student Address: " + student.getStudentAddress());
}
}
```
在上述示例代码中,我们创建了一个名为 `student` 的 `Student` 对象,并使用构造方法来初始化其属性值。然后,我们使用相应的 Getter 方法来获取这些属性的值,并将其输出到控制台上。
阅读全文
相关推荐
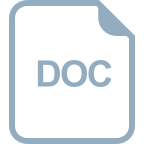
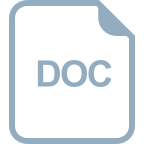
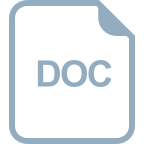
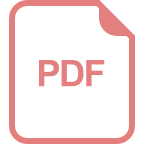














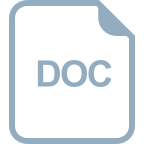