python读取txt
时间: 2023-06-23 13:55:19 浏览: 38
要读取一个txt文件,可以使用Python内置的`open()`函数来打开文件,然后使用`.read()`或`.readlines()`方法来读取文件内容。例如:
```python
# 打开文件
file = open('file.txt', 'r')
# 读取整个文件内容
content = file.read()
print(content)
# 读取每一行内容
lines = file.readlines()
for line in lines:
print(line)
# 关闭文件
file.close()
```
请注意,`open()`函数第一个参数是文件名,第二个参数是文件打开模式。`'r'`表示只读模式,如果文件不存在,会抛出`FileNotFoundError`异常。在读取完文件后,一定要记得关闭文件,可以使用`close()`方法来关闭。
相关问题
python 读取 txt
以下是Python读取txt文件的方法:
1. 使用with语句打开文件,可以自动关闭文件,避免资源泄露。使用read()方法读取整个文件内容。
```python
with open('file.txt', 'r') as f:
content = f.read()
print(content)
```
2. 使用with语句打开文件,使用readline()方法逐行读取文件内容。
```python
with open('file.txt', 'r') as f:
line = f.readline()
while line:
print(line)
line = f.readline()
```
3. 使用with语句打开文件,使用readlines()方法读取整个文件内容,并将其存储在列表中。
```python
with open('file.txt', 'r') as f:
lines = f.readlines()
for line in lines:
print(line)
```
python读写txt
Python提供了内置的文件操作功能,可以方便地读写.txt格式的文本文件。以下是如何在Python中进行读写操作的简单示例:
### 读取txt文件:
```python
# 打开文件(如果不存在会自动创建)
with open('example.txt', 'r') as file:
# 读取整个文件内容
content = file.read()
# 或者逐行读取
lines = file.readlines()
# 打印内容或做其他处理
print(content)
```
### 写入txt文件:
```python
# 写入内容
with open('example.txt', 'w') as file:
file.write('这是写入的内容\n')
# 可以使用file对象的write方法多次写入
# 如果要追加内容而不是覆盖原有内容,使用'a'模式
with open('example.txt', 'a') as file:
file.write('这是追加的内容\n')
```
### 相关问题--:
1. Python中的文件操作模式有哪些?
2. 如何处理读取大文件,避免一次性加载到内存中?
3. Python如何保证写入txt文件的字符编码正确性?
相关推荐
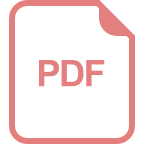
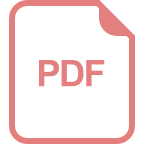
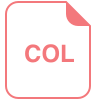
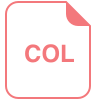
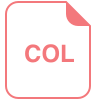
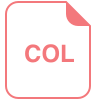
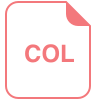






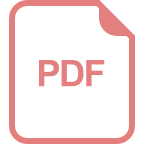