opencv C++ 调用opencv自带的文字图片
时间: 2024-07-29 15:01:42 浏览: 54
在 OpenCV 中,如果你想要加载并操作包含文字的图像(通常是位图或矢量格式),你可以使用 `imread()` 函数来读取图像文件,然后配合 `getText()` 或者 `findContours()` 等方法来识别文本。这里以读取位图图像为例:
```cpp
#include <opencv2/opencv.hpp>
int main() {
// 加载图片
cv::Mat img = cv::imread("path_to_your_text_image.jpg", cv::IMREAD_COLOR);
if (img.empty()) {
std::cout << "Could not open or find the image." << std::endl;
return -1;
}
// 如果需要检测和识别文本,可以尝试以下步骤:
// 将图像转换为灰度图像
cv::Mat grayImg;
cv::cvtColor(img, grayImg, cv::COLOR_BGR2GRAY);
// 应用边缘检测(如Canny算子)
cv::Canny(grayImg, grayImg, 50, 150);
// 寻找轮廓(可能包含文本区域)
std::vector<std::vector<cv::Point>> contours;
cv::findContours(grayImg, contours, cv::RETR_EXTERNAL, cv::CHAIN_APPROX_SIMPLE);
for (const auto& contour : contours) {
// 对每个轮廓进行进一步处理,比如 OCR(光学字符识别)
cv::Rect boundingBox = cv::boundingRect(contour);
cv::rectangle(img, boundingBox, cv::Scalar(0, 0, 255), 2); // 绘制边界框
}
// 显示处理后的图像
cv::imshow("Detected Text", img);
cv::waitKey(0);
return 0;
}
```
在这个例子中,我们首先读取图像,然后将其转换为灰度并应用边缘检测。之后,我们找到轮廓并检测可能的文本区域。注意,这只是一个基础示例,实际的文本识别通常会更复杂,涉及到专门的库,如 Tesseract OCR。
阅读全文
相关推荐
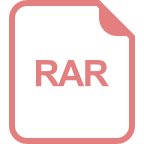
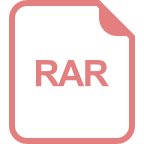
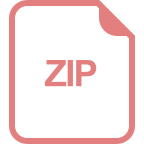
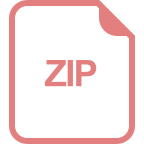
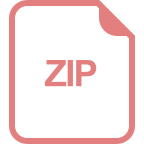
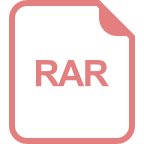
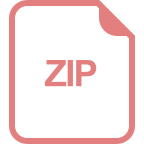
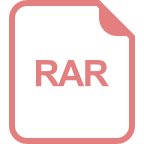
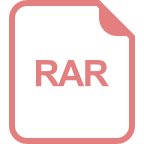
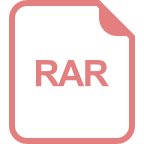
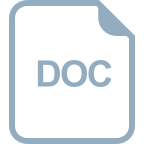
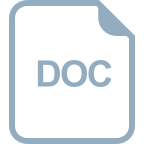
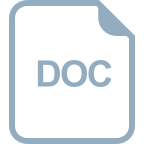