LinkList * SetNull LinkList(LinkList * head) while (head->next) Delete After_LinkList (head) ; return head:
时间: 2024-09-11 11:17:41 浏览: 42
要实现`SetNull LinkList(LinkList * head)`这个功能,我们需要创建一个新的函数来置链表头节点的下一个指针为空。这实际上就是删除链表的第一个元素(如果存在),使其成为一个空链表。下面是一个简单的步骤描述:
1. 定义一个新的函数`SetNull(LinkList * head)`,它接受一个链表头节点作为参数。
2. 使用一个`while`循环来遍历链表,直到`head->next`为空(即链表结束)。
3. 在循环内部,调用`DeleteAfterLinkList(head)` 函数,该函数会删除`head`的下一个节点[^2],并将`head`移动到下一个节点的位置。
4. 循环结束后,`head`将指向原链表的第一个节点,但由于我们已经删除了所有后续节点,所以`head->next`现在是`NULL`。
5. 返回`head`,它现在是新链表的头,也是一个空链表的头。
```c++
// 假设 DeleteAfterLinkList 是一个已有的函数用于删除一个节点
LinkList * SetNull(LinkList * head) {
while (head->next != NULL) { // 当还有其他节点时
DeleteAfterLinkList(head); // 删除下一个节点
head = head->next; // 更新 head 指向下一个节点
}
return head; // 返回新的头节点,此时链表为空
}
```
相关问题
修改下列代码要求如下:每组数据输出n-1行。为删除顶点后的邻接表。每两个数字之间用空格隔开。代码如下:#include<iostream> #include<string> #include<algorithm> #include<vector> #include<set> #include<map> using namespace std;typedef struct LNode {int data;struct LNode* next; }*linklist, LNode; typedef struct {int vexnum;int arcnum;linklist VList; }ALGragh; void CreateUDG(ALGragh& G, int n, int m) {G.arcnum = m;G.vexnum = n;G.VList = new LNode[n + 1];for (int i = 1; i <= n; i++){G.VList[i].data = i;G.VList[i].next = NULL;}int h, k;for (int i = 0; i < m; i++){cin >> h >> k;linklist p = new LNode, q = new LNode;p->data = h;p->next = G.VList[k].next;G.VList[k].next = p;q->data = k;q->next = G.VList[h].next;G.VList[h].next = q;} } void PrintGraph(ALGragh G) {for (int i = 1; i <= G.vexnum; i++){linklist p = &G.VList[i];while (p->next){cout << p->data << ' ';p = p->next;}cout << p->data << endl;} } void DeleteVex(ALGragh& G) {int h, k;cin >> h >> k;for (int i = 1; i <= G.vexnum ; i++){linklist p = &G.VList[i];if (i == h){if (p->next){if (p->next->data == k)p->next = p->next->next;}}if (i == k){if (p->next){if (p->next->data == h)p->next = p->next->next;}}} }
修改后的代码如下所示:
```
#include<iostream>
#include<string>
#include<algorithm>
#include<vector>
#include<set>
#include<map>
using namespace std;
typedef struct LNode {
int data;
struct LNode* next;
}*linklist, LNode;
typedef struct {
int vexnum;
int arcnum;
linklist VList;
}ALGragh;
void CreateUDG(ALGragh& G, int n, int m) {
G.arcnum = m;
G.vexnum = n;
G.VList = new LNode[n + 1];
for (int i = 1; i <= n; i++){
G.VList[i].data = i;
G.VList[i].next = NULL;
}
int h, k;
for (int i = 0; i < m; i++){
cin >> h >> k;
linklist p = new LNode, q = new LNode;
p->data = h;
p->next = G.VList[k].next;
G.VList[k].next = p;
q->data = k;
q->next = G.VList[h].next;
G.VList[h].next = q;
}
}
void PrintGraph(ALGragh G) {
for (int i = 1; i <= G.vexnum; i++){
linklist p = &G.VList[i];
while (p->next){
cout << p->data << ' ';
p = p->next;
}
cout << p->data << endl;
}
}
void DeleteVex(ALGragh& G) {
int h, k;
cin >> h >> k;
linklist p = G.VList[h].next;
while (p) {
if (p->data == k) {
G.VList[h].next = p->next;
break;
}
p = p->next;
}
p = G.VList[k].next;
while (p) {
if (p->data == h) {
G.VList[k].next = p->next;
break;
}
p = p->next;
}
}
int main() {
int n, m;
cin >> n >> m;
ALGragh G;
CreateUDG(G, n, m);
for (int i = 1; i <= n; i++) {
ALGragh temp = G;
DeleteVex(temp);
PrintGraph(temp);
}
return 0;
}
```
修改后的代码中,新增了一个 `main()` 函数,其中包含了循环删除每个顶点的代码。在 `DeleteVex()` 函数中,修改了删除顶点的逻辑,使其可以正确删除指定顶点,并且同时更新相邻顶点的邻接表。在每次删除顶点后,调用 `PrintGraph()` 函数输出邻接表即可。
*/ /*尾插法建立单链表*/ LinkList CreateList(int n) { LinkList head, s, p; head = (LinkList)malloc(sizeof(LNode)); head->next = NULL; p = head; return head; }
To implement the tail insertion method for creating a singly linked list in C, you can use the following code:
typedef struct LNode{
int data;
struct LNode *next;
}LNode, *LinkList;
LinkList CreateList(int n) {
LinkList head, s, p; // declare three pointers: head, s, and p
head = (LinkList)malloc(sizeof(LNode)); // allocate memory for head
head->next = NULL; // set the next pointer of head to NULL
p = head; // let p point to head initially
int i;
for(i=1; i<=n; i++) {
s = (LinkList)malloc(sizeof(LNode)); // allocate memory for the new node
printf("Please enter the data of node %d: ", i);
scanf("%d", &(s->data)); // read the data for the new node
s->next = NULL; // set the next pointer to NULL
p->next = s; // insert the new node after the last node
p = s; // let p point to the last node now
}
return head; // return the head pointer of the new linked list
}
In the above code, you first define the structure of a singly linked list and two pointers: head and p. Then, you allocate memory for the head node and set its next pointer to NULL. After that, you loop through n times to create n new nodes. For each new node, you allocate memory for it, read its data from user input, set its next pointer to NULL, and insert it after the last node of the current list. Finally, you return the head pointer of the new linked list.
阅读全文
相关推荐
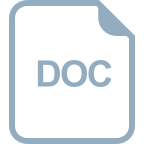
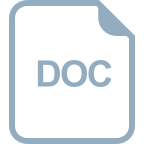
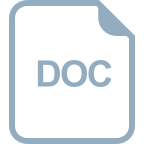

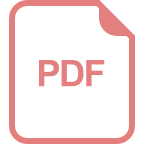
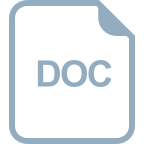
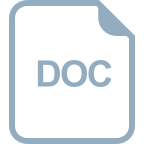



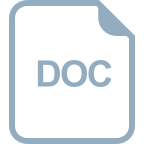
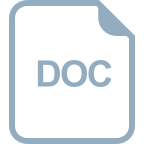
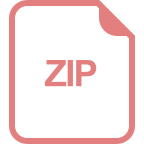
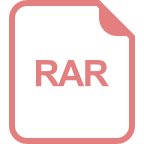
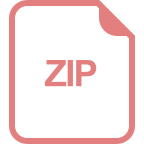