在右侧编辑器自行定义数据类型,实现两个矩阵的相加。 要求: 1、当两个输入矩阵的行数或列数不同时,认为两个矩阵无法进行相加,输出0。 2、当两个矩阵进行相加时,若相加后产生0的结果,则不计入TSMatrix中的data数组中。 3、注意每行输出后都有一个空格。
时间: 2024-12-18 17:34:36 浏览: 17
在C语言中,我们可以定义一个自定义的数据类型来表示矩阵(TSMatrix),并实现矩阵相加的功能。首先,我们需要为矩阵定义一个结构体,包含行数、列数以及数据数组。如果矩阵的大小不同,我们将返回一个特殊的值来表示无法相加。
```c
#include <stdio.h>
// 自定义矩阵类型定义
typedef struct {
int rows;
int cols;
int data[100][100]; // 假设矩阵最大是100x100,实际应用可能需要更大的数组
} TSMatrix;
// 检查矩阵是否可以相加
int can_add(const TSMatrix* mat1, const TSMatrix* mat2) {
return mat1->rows == mat2->rows && mat1->cols == mat2->cols;
}
// 矩阵相加
void matrix_add(TSMatrix* result, const TSMatrix* mat1, const TSMatrix* mat2) {
if (!can_add(mat1, mat2)) {
printf("0\n"); // 如果行列数不同,输出0
return;
}
for (int i = 0; i < result->rows; ++i) {
for (int j = 0; j < result->cols; ++j) {
result->data[i][j] = mat1->data[i][j] + mat2->data[i][j];
if (result->data[i][j] == 0) continue; // 不计入结果,避免0元素
printf("%d ", result->data[i][j]);
}
printf("\n");
}
}
int main() {
TSMatrix mat1 = {5, 5, {0}}, mat2 = {5, 5, {0}}; // 初始化矩阵
TSMatrix result;
// 进行矩阵相加
matrix_add(&result, &mat1, &mat2);
return 0;
}
```
阅读全文
相关推荐

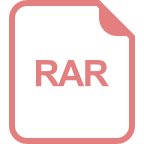
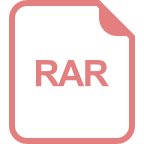















