水温水位在线监测系统数据后台接收服务程序代码,用Java,数据库用MySQL
时间: 2024-09-27 21:02:19 浏览: 36
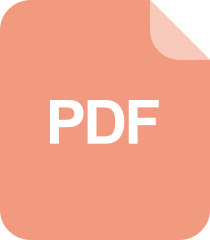
论文研究-嵌入式水温水位监测系统的设计与应用 .pdf
在Java中创建一个用于接收水温水位在线监测系统数据并存储到MySQL数据库的后台服务程序,通常会涉及到以下几个步骤:
1. **环境配置**:
- 首先,你需要设置好Java开发环境,比如JDK、Eclipse/IntelliJ IDEA等IDE。
- 安装MySQL JDBC驱动(`mysql-connector-java`),以便连接数据库。
2. **数据库连接**:
```java
String url = "jdbc:mysql://localhost:3306/your_database_name";
String username = "your_username";
String password = "your_password";
try (Connection conn = DriverManager.getConnection(url, username, password)) {
// 数据库连接成功后可以继续操作
} catch (SQLException e) {
e.printStackTrace();
}
```
3. **定义数据模型**:
对于水温和水位的数据,可能会有对应的实体类(如WaterLevelData)表示。
4. **创建服务接收数据**:
```java
@PostMapping("/data")
public void receiveData(@RequestBody WaterLevelData data) {
try {
// 将接收到的数据保存到数据库
saveToDatabase(data);
} catch (Exception e) {
log.error("Failed to save data", e);
}
}
private void saveToDatabase(WaterLevelData data) throws SQLException {
String sql = "INSERT INTO water_levels (temperature, level) VALUES (?, ?)";
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setDouble(1, data.getTemperature());
pstmt.setDouble(2, data.getLevel());
pstmt.executeUpdate();
}
```
5. **异常处理**:
确保对可能出现的网络中断、数据库连接错误等异常进行适当的处理。
```java
// 使用Spring Boot可以简化数据访问和异常处理
@SpringBootApplication
public class MonitoringServerApplication {
public static void main(String[] args) {
SpringApplication.run(MonitoringServerApplication.class, args);
}
@Autowired
private DataSource dataSource;
@ExceptionHandler(SQLException.class)
public ResponseEntity<String> handleSqlException(SQLException ex) {
return new ResponseEntity<>("Error saving data: " + ex.getMessage(), HttpStatus.INTERNAL_SERVER_ERROR);
}
}
```
阅读全文
相关推荐
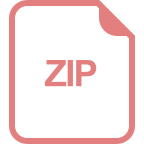
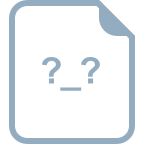
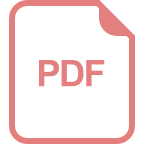
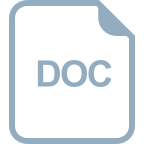
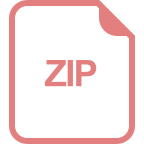
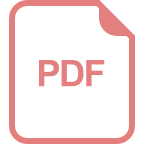
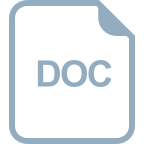
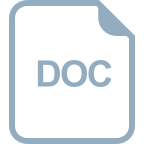
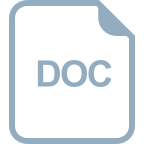
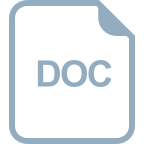
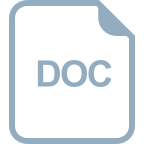
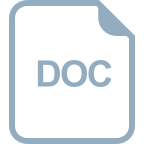


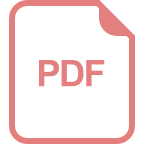