Linux中打开一个文件夹,删除里面的TXT文件的C语言代码
时间: 2024-10-23 22:10:52 浏览: 31
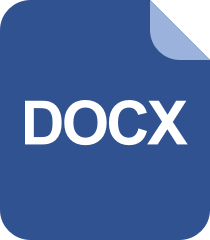
Linux快捷键以及使用,C语言学习笔记
在Linux环境下,如果你想用C语言编写程序来遍历目录并删除所有的TXT文件,可以使用`<dirent.h>`头文件来处理目录和`<unistd.h>`头文件来执行文件操作。下面是一个简单的示例,它会递归地查找指定目录下的所有TXT文件并删除它们:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <dirent.h>
#include <sys/stat.h>
#include <unistd.h>
void delete_txt_files(const char *dir_path) {
struct dirent *entry;
DIR *dp = opendir(dir_path);
if (dp == NULL) {
perror("Error opening directory");
return;
}
while ((entry = readdir(dp)) != NULL) {
if (strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0) {
continue; // Skip '.' and '..'
}
char full_path[PATH_MAX];
snprintf(full_path, sizeof(full_path), "%s/%s", dir_path, entry->d_name);
struct stat file_stat;
if (stat(full_path, &file_stat) == -1) {
perror("Error getting file stats");
continue;
}
if (S_ISREG(file_stat.st_mode) && strstr(entry->d_name, ".txt")) { // Check if it's a regular file and ends with .txt
if (unlink(full_path) == -1) {
perror("Error deleting file");
} else {
printf("Deleted file: %s\n", full_path);
}
}
}
closedir(dp);
}
int main(int argc, char **argv) {
if (argc != 2) {
fprintf(stderr, "Usage: %s <directory_path>\n", argv[0]);
return 1;
}
const char *dir_path = argv[1];
delete_txt_files(dir_path);
return 0;
}
```
要运行这个程序,你需要在命令行输入 `./your_program_name /path/to/directory`,其中`your_program_name`是你的程序名称,`/path/to/directory`是你想要搜索和删除TXT文件的目录路径。
注意:这个程序直接删除文件,所以在实际应用中需要谨慎处理,特别是在生产环境中。此外,权限问题也需要考虑。
阅读全文
相关推荐
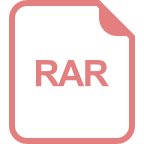
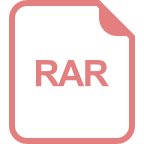
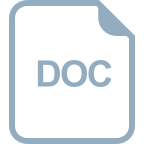
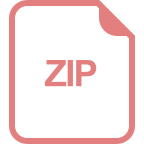
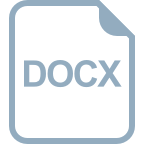
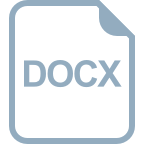
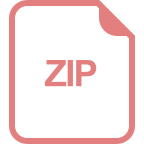
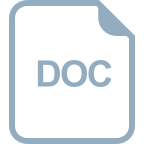
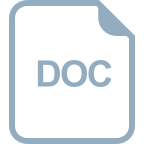
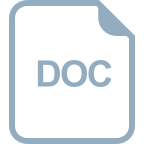
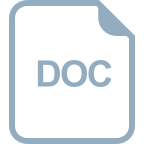
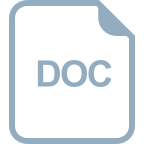
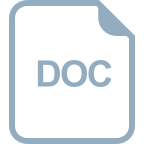
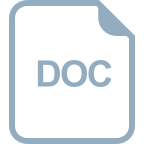
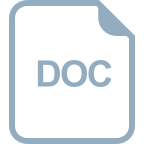
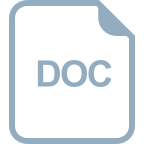
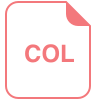
