from IPython.display import clear_output, Image import time import cv2 from PIL import Image as PILImage import io current_time = 0 def processImg(img): # 画出一个框 cv2.rectangle(img, (500, 300), (800,400), (0, 0, 255), 5) # 显示FPS global current_time if current_time == 0: current_time = time.time() else: last_time = current_time current_time = time.time() fps = 1. / (current_time - last_time) text = "FPS: %d" % int(fps) cv2.putText(img, text, (0, 100), cv2.FONT_HERSHEY_TRIPLEX, 3.65, (255, 0, 0), 2) return img def arrayShow(imageArray): pil_image = PILImage.fromarray(imageArray) # 将图像转换为字节数据 io.BytesIO() pil_image.save(stream, format='PNG') display(Image(stream.getvalue())) video_url = "http://192.168.50.180/mjpeg/1" video = cv2.VideoCapture(video_url) while True: try: clear=True ret, frame = video.read() if not ret: break lines, columns, _ = frame.shape frame = processImg(frame) frame = cv2.resize(frame, (int(columns / 4), int(lines / 4))) arrayShow(frame) time.sleep(0.02) except KeyboardInterrupt: video.release()帮我修改一下这份代码
时间: 2024-04-19 14:24:40 浏览: 184
好的,我注意到你想要在Jupyter Notebook中显示实时视频流,并在视频帧上绘制一个框和FPS信息。以下是修改后的代码:
```python
from IPython.display import clear_output, Image
import time
import cv2
from PIL import Image as PILImage
import io
current_time = 0
def processImg(img):
# 绘制一个框
cv2.rectangle(img, (500, 300), (800,400), (0, 0, 255), 5)
# 显示FPS
global current_time
if current_time == 0:
current_time = time.time()
else:
last_time = current_time
current_time = time.time()
fps = 1. / (current_time - last_time)
text = "FPS: %d" % int(fps)
cv2.putText(img, text, (0, 100), cv2.FONT_HERSHEY_TRIPLEX, 3.65, (255, 0, 0), 2)
return img
def arrayShow(imageArray):
pil_image = PILImage.fromarray(imageArray)
# 将图像转换为字节数据
stream = io.BytesIO()
pil_image.save(stream, format='PNG')
display(Image(stream.getvalue()))
video_url = "http://192.168.50.180/mjpeg/1"
video = cv2.VideoCapture(video_url)
try:
while True:
clear=True
ret, frame = video.read()
if not ret:
break
lines, columns, _ = frame.shape
frame = processImg(frame)
frame = cv2.resize(frame, (int(columns / 4), int(lines / 4)))
arrayShow(frame)
time.sleep(0.02)
except KeyboardInterrupt:
video.release()
clear_output()
```
这个修改后的代码修复了几个问题:
1. 在`arrayShow()`函数中,修复了保存图像字节数据的错误。添加了缺少的`stream`变量,并将其传递给`Image()`函数。
2. 在主循环中,添加了对`KeyboardInterrupt`异常的处理,以便在按下键盘中断时正确释放视频捕获对象,并清除输出。
请尝试运行修改后的代码,看看是否能够正常显示视频流并绘制框和FPS信息。如果还有其他问题,请提供更多信息,我会尽力帮助你解决。
阅读全文
相关推荐
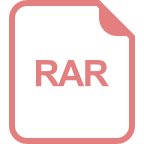
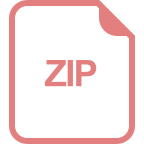
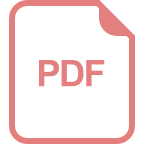
















