涉及代码思路解析最好给出代码
时间: 2024-12-25 15:24:12 浏览: 14
理解您的需求后,我将对论文中的算法进行简要解析,并尝试提供一个简化版的Python代码示例来展示其核心思想。请注意,由于实际应用中需要处理复杂的数学运算和物理模型,以下代码仅用于演示目的,并未完全实现所有功能。
### 论文核心思想解析
论文提出了一种高效的递进算法(Progressive Algorithm),用于计算多层同心纳米粒子的光散射特性。该算法的主要特点包括:
1. **逐层递进**:从内核开始,逐步向最外层推进,每次只考虑相邻两层之间的相互作用。
2. **矩阵乘法**:在每一步中,通过4×4矩阵的操作来更新散射参数,而不是一次性求解大规模线性方程组。
3. **选择性计算**:可以单独计算任意一层的散射系数,而不需要计算整个粒子的所有层。
### 核心公式
假设我们有两个相邻的壳层 \( l \) 和 \( l+1 \),它们的电场和磁场可以用多极展开表示为:
\[ E_l = \sum_{n=1}^{\infty} \sum_{m=-n}^{n} E_n \left( c_{mn}^l M_{mn}^{(1)} + d_{mn}^l N_{mn}^{(1)} + b_{mn}^l M_{mn}^{(3)} + a_{mn}^l N_{mn}^{(3)} \right) \]
\[ E_{l+1} = \sum_{n=1}^{\infty} \sum_{m=-n}^{n} E_n \left( c_{mn}^{l+1} M_{mn}^{(1)} + d_{mn}^{l+1} N_{mn}^{(1)} + b_{mn}^{l+1} M_{mn}^{(3)} + a_{mn}^{l+1} N_{mn}^{(3)} \right) \]
边界条件要求在两个层之间的界面上,切向电场和磁场连续,即:
\[ (E_{l+1} - E_l) \times \hat{e}_r = 0 \]
\[ (H_{l+1} - H_l) \times \hat{e}_r = 0 \]
这些条件可以转化为四个方程,最终形成一个4×4矩阵方程:
\[ [M]_l \begin{pmatrix} a_{l+1} \\ b_{l+1} \\ c_{l+1} \\ d_{l+1} \end{pmatrix} - [N]_l \begin{pmatrix} a_l \\ b_l \\ c_l \\ d_l \end{pmatrix} = 0 \]
通过矩阵操作,可以得到:
\[ \begin{pmatrix} a_{l+1} \\ b_{l+1} \\ c_{l+1} \\ d_{l+1} \end{pmatrix} = [P]_l \begin{pmatrix} a_l \\ b_l \\ c_l \\ d_l \end{pmatrix} \]
其中,\[ [P]_l = [M]_l^{-1} [N]_l \]
### Python 示例代码
以下是一个简化的Python代码示例,展示了如何使用4×4矩阵逐步计算多层粒子的散射系数。
```python
import numpy as np
def riccati_bessel_functions(n, x):
""" 计算Riccati-Bessel函数 """
psi = x * np.spherical_jn(n, x)
xi = x * (np.spherical_jn(n, x) + 1j * np.spherical_yn(n, x))
return psi, xi
def calculate_P_matrix(n, m, x, m_next, x_next):
""" 计算P矩阵 """
psi, xi = riccati_bessel_functions(n, x)
psi_next, xi_next = riccati_bessel_functions(n, x_next)
det = m * xi_next * psi - m_next * xi * psi_next
p11 = (m_next * xi_next * psi - m * xi * psi_next) / det
p14 = (m_next * psi_next * psi - m * psi * psi_next) / det
p22 = (m * xi_next * psi - m_next * xi * psi_next) / det
p23 = (m * psi_next * psi - m_next * psi * psi_next) / det
p32 = (m_next * xi_next * xi - m * xi * xi_next) / det
p33 = (m_next * xi_next * psi - m * xi * psi_next) / det
p41 = (m * xi_next * xi - m_next * xi * xi_next) / det
p44 = (m * xi_next * psi - m_next * xi * psi_next) / det
P = np.array([[p11, 0, 0, p14],
[0, p22, p23, 0],
[0, p32, p33, 0],
[p41, 0, 0, p44]])
return P
def progressive_algorithm(layers, k, m_values):
""" 递进算法主函数 """
# 初始化内核的散射系数
a_core = 0
b_core = 0
c_core = 0
d_core = 0
# 从内核到最外层逐层计算
for i in range(len(layers) - 1):
r_i = layers[i]
r_next = layers[i + 1]
m_i = m_values[i]
m_next = m_values[i + 1]
x = k * r_i
x_next = k * r_next
P_matrix = calculate_P_matrix(1, m_i, x, m_next, x_next)
# 更新当前层的散射系数
a_next = P_matrix[0, 0] * a_core + P_matrix[0, 3] * d_core
b_next = P_matrix[1, 1] * b_core + P_matrix[1, 2] * c_core
c_next = P_matrix[2, 1] * b_core + P_matrix[2, 2] * c_core
d_next = P_matrix[3, 0] * a_core + P_matrix[3, 3] * d_core
# 更新内核的散射系数
a_core = a_next
b_core = b_next
c_core = c_next
d_core = d_next
return a_core, b_core, c_core, d_core
# 示例数据
layers = [40, 60, 90] # 各层半径
k = 1.0 # 波数
m_values = [1.0, 1.5, 1.0] # 各层折射率
# 调用递进算法
a, b, c, d = progressive_algorithm(layers, k, m_values)
print("最终散射系数:")
print(f"a: {a}, b: {b}, c: {c}, d: {d}")
```
### 解释
1. **Riccati-Bessel 函数**:`riccati_bessel_functions` 函数计算Riccati-Bessel函数,这是解决电磁散射问题的关键函数。
2. **P 矩阵计算**:`calculate_P_matrix` 函数根据给定的层数、半径和折射率计算P矩阵。
3. **递进算法主函数**:`progressive_algorithm` 函数从内核开始,逐步向外层推进,更新每一层的散射系数。
这个示例代码展示了如何使用4×4矩阵逐步计算多层粒子的散射系数。实际应用中可能需要更复杂的数学运算和优化,但基本思路是一致的。希望这对您有所帮助!
阅读全文
相关推荐









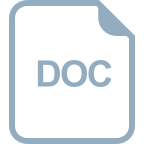
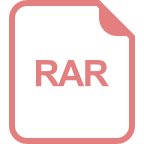







