spring boot 图片上传服务器
时间: 2023-08-01 19:11:01 浏览: 54
在Spring Boot中实现图片上传到服务器可以通过以下步骤完成:
1. 添加依赖:在pom.xml文件中添加以下依赖,以支持文件上传功能:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.4</version>
</dependency>
```
2. 配置文件上传路径:在application.properties或application.yml文件中配置上传文件的保存路径。例如:
```yaml
spring:
servlet:
multipart:
enabled: true
location: /path/to/save/files
```
3. 创建文件上传接口:创建一个Controller类,用于处理文件上传的请求。示例代码如下:
```java
import org.springframework.beans.factory.annotation.Value;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import java.io.File;
import java.io.IOException;
@RestController
public class FileUploadController {
@Value("${spring.servlet.multipart.location}")
private String uploadDir;
@PostMapping("/upload")
public ResponseEntity<String> uploadFile(@RequestParam("file") MultipartFile file) {
try {
String fileName = file.getOriginalFilename();
String filePath = uploadDir + File.separator + fileName;
file.transferTo(new File(filePath));
return ResponseEntity.ok("File uploaded successfully!");
} catch (IOException e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("Failed to upload file!");
}
}
}
```
4. 启动应用程序:运行Spring Boot应用程序并访问`http://localhost:8080/upload`,选择要上传的图片文件,然后点击上传按钮即可将图片上传到服务器指定的路径。
请注意,上述示例中的路径`/path/to/save/files`需要根据实际情况进行更改,并确保你有权限在该路径下创建文件。
相关推荐
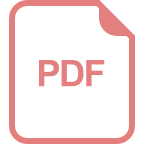














