python QT 打开网址
时间: 2023-08-19 10:00:14 浏览: 43
你可以使用 PyQt 或 PySide 库来在 Python 中使用 Qt 框架打开网址。以下是一个示例代码:
```python
import sys
from PyQt5.QtCore import QUrl
from PyQt5.QtWidgets import QApplication
from PyQt5.QtWebEngineWidgets import QWebEngineView
app = QApplication(sys.argv)
web_view = QWebEngineView()
web_view.load(QUrl("https://www.example.com"))
web_view.show()
sys.exit(app.exec_())
```
上述代码使用了 PyQt5 和 QtWebEngine 模块来创建一个带有 Web 视图的简单应用程序。在 `load()` 方法中,你可以传入你想要打开的网址。运行上述代码将会打开一个窗口并显示指定的网址内容。记得在运行之前安装相应的库。
相关问题
Python qt 打开可执行程序
可以使用Python中的`subprocess`模块来打开可执行程序。以下是一个简单的例子:
```
import subprocess
# 打开可执行程序
subprocess.call('./executable', shell=True)
```
其中`./executable`是你要打开的可执行程序的路径。如果你不知道可执行程序的完整路径,可以使用`os.path`模块来获取。
Python qt 点击按钮 打开文件
您好,对于您的问题,您可以使用Python中的Qt库来实现点击按钮打开文件的功能。以下是一个简单的示例代码:
``` python
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QFileDialog
class Example(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setGeometry(300, 300, 300, 220)
self.setWindowTitle('打开文件')
self.btn = QPushButton('打开文件', self)
self.btn.move(20, 70)
self.btn.clicked.connect(self.showDialog)
self.show()
def showDialog(self):
fname = QFileDialog.getOpenFileName(self, 'Open file', '/home')
if fname[0]:
print(fname[0])
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = Example()
sys.exit(app.exec_())
```
在这个代码中,我们首先导入了需要用到的库。然后我们定义了一个名为Example的窗口,并在该窗口中添加了一个名为btn的按钮。当我们点击这个按钮时,会调用showDialog方法。在这个方法中,我们通过QFileDialog来打开文件,并将文件路径打印出来。
希望这个代码可以帮助到您。如果您有任何其他问题,请随时问我。
相关推荐
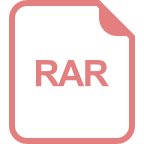
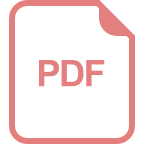












