如何在Python中实现十折交叉验证(Ten-Fold Cross Validation)?
时间: 2024-11-09 11:21:09 浏览: 99
在Python中,我们可以使用sklearn库来方便地实现十折交叉验证。以下是一个简单的步骤:
1. 首先,导入所需的模块:
```python
from sklearn.model_selection import KFold
from sklearn.linear_model import LogisticRegression
from sklearn.datasets import load_iris
```
2. 加载数据集,这里我们以Iris数据集为例:
```python
iris = load_iris()
X = iris.data
y = iris.target
```
3. 创建一个KFold实例,指定分组数(这里是10):
```python
kfold = KFold(n_splits=10, shuffle=True, random_state=42)
```
`shuffle=True`确保每次划分数据时都打乱顺序,`random_state`用于保持拆分结果的一致性。
4. 对每个训练集和测试集执行模型训练和评估:
```python
model = LogisticRegression() # 或者你喜欢的其他模型
scores = []
for train_index, val_index in kfold.split(X):
X_train, X_val = X[train_index], X[val_index]
y_train, y_val = y[train_index], y[val_index]
model.fit(X_train, y_train)
score = model.score(X_val, y_val)
scores.append(score)
```
5. 最后,你可以计算平均得分,评估模型的整体性能:
```python
average_score = sum(scores) / len(scores)
print(f"Average cross-validation score: {average_score}")
```
这就是在Python中使用sklearn实现十折交叉验证的基本流程。
阅读全文
相关推荐
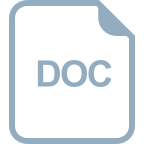
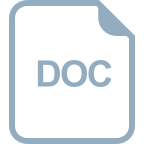
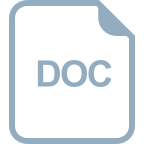
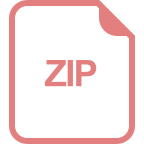
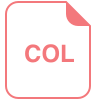

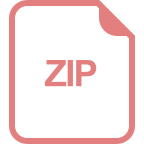
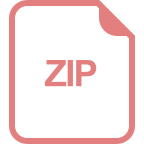
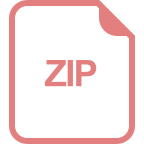
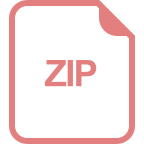








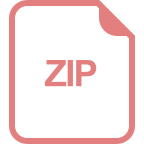