如何在.NET中检查HTTP请求的身份验证?
时间: 2024-10-23 11:12:23 浏览: 14
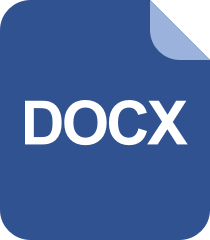
基于ASP.NET MVC3的身份验证及权限控制方案设计
在.NET中,`HttpListener`默认不支持内置的身份验证,你需要手动配置或使用第三方库来处理HTTP身份验证。以下是一个简单的示例,展示如何使用自定义Basic身份验证:
1. **创建自定义验证器**:
```csharp
public class CustomAuthenticationHandler : HttpListenerContextWrapper, IAuthenticationHandler
{
public bool Authenticate(string userName, string password)
{
// 实现你的用户名密码验证逻辑
if (userName == "admin" && password == "password") return true; // 示例验证
return false;
}
}
```
2. **设置身份验证**:
```csharp
HttpListener listener = new HttpListener();
listener.AuthenticationSchemes = AuthenticationSchemes.Basic;
listener.UseCredentials = true;
listener.Context.AuthenticationMethods.Clear();
listener.Context.AuthenticationMethods.Add(AuthenticationSchemes.Basic);
listener.Context.AuthenticationHandlers.Add(new CustomAuthenticationHandler());
```
3. **处理未经验证的请求**:
```csharp
listener.BeginGetContext(asyncResult =>
{
try
{
var context = await listener.EndGetContext(asyncResult);
if (!context.User.Identity.IsAuthenticated) // 检查是否已通过验证
{
context.Response.StatusCode = 401; // 发送未授权状态码
context.Response.Headers["WWW-Authenticate"] = "Basic realm=\"Your Realm\"";
context.Response.Close();
}
else
{
// 请求经过验证,继续正常处理
}
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
}
}, null);
```
当客户端发起请求时,如果你的验证失败,会返回401未经授权的状态码并附带适当的`WWW-Authenticate`头部,提示需要提供正确的凭据。
阅读全文
相关推荐
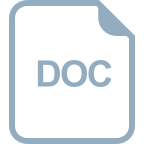
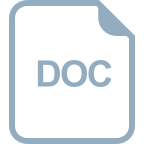
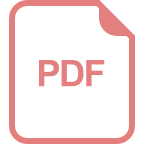
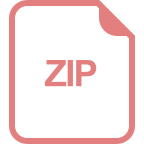
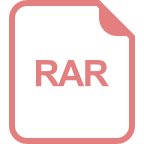
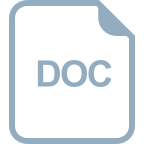
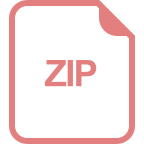
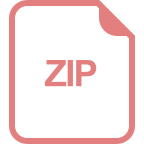
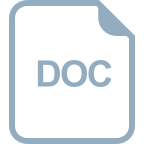
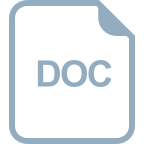
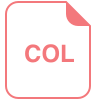
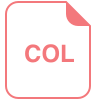
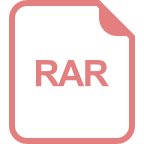
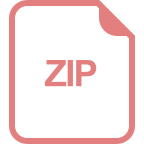
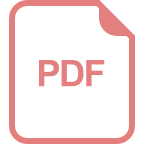
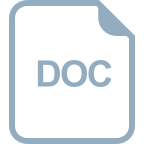
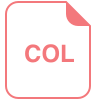
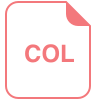