如何使用Python编程语言构建一个模拟沙漠寻宝的算法,其中宝藏位置由一个二维数组表示,目标是找到从起点到宝藏的最短路径并可视化为流程图?
时间: 2024-10-23 20:18:20 浏览: 45
在Python中,你可以使用`pygame`库来构建一个简单的图形界面,并结合`networkx`库处理寻路算法,如Dijkstra或A*搜索。首先,你需要安装这两个库,通过命令行运行以下命令:
```
pip install pygame networkx
```
接下来,这里有一个基础的框架,演示如何使用Dijkstra算法找到最短路径并在流程图上可视化:
```python
import pygame
import networkx as nx
from pygame.locals import *
# 定义地图(二维数组)
map_data = [[0 for _ in range(10)] for _ in range(10)] # 初始化一个空地图
start_node = (0, 0)
end_node = (9, 9)
# 构建图
graph = nx.Graph()
for i in range(len(map_data)):
for j in range(len(map_data[i])):
graph.add_node((i, j), weight=map_data[i][j]) # 如果该位置是障碍物,则weight设为无穷大
graph.add_edge(start_node, end_node, weight=0) # 确保起始点和终点相连
def dijkstra_search(graph, start, end):
path = nx.dijkstra_path(graph, start, end)
return path
# 寻找最短路径
path = dijkstra_search(graph, start_node, end_node)
# 初始化Pygame
pygame.init()
# 绘制地图和路径
screen = pygame.display.set_mode((800, 600))
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 在屏幕绘制地图和路径
draw_map(screen, graph, path)
pygame.display.flip()
def draw_map(surface, graph, path):
for node in path:
x, y = node
color = (0, 255, 0) if node in [start_node, end_node] else (255, 255, 255)
pygame.draw.rect(surface, color, (x * 80, y * 80, 80, 80))
# 结束程序
pygame.quit()
```
阅读全文
相关推荐
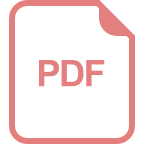
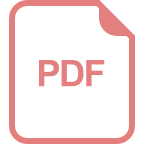
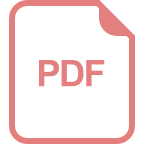
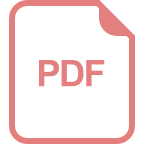
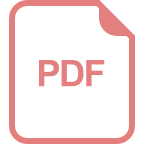
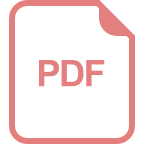
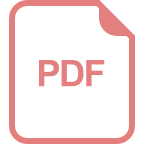
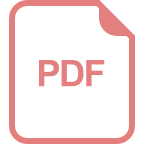
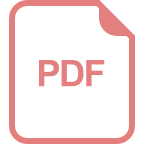
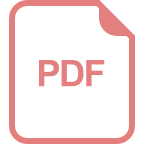
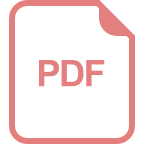
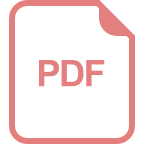
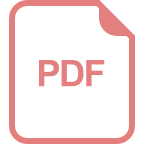
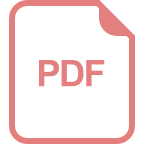
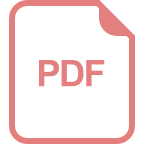
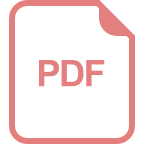
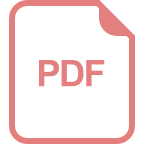
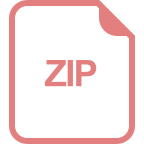
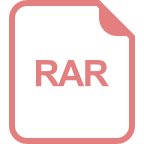