jsp+MySQL写加入购物车
时间: 2023-08-01 21:15:37 浏览: 64
好的,这是一个典型的电商应用场景,下面是一个简单的jsp+MySQL实现加入购物车的例子。
首先,我们需要设计一个商品列表页面,展示所有的商品信息,每个商品都有一个对应的添加到购物车的按钮。
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>商品列表</title>
</head>
<body>
<h1>商品列表</h1>
<table>
<tr>
<th>商品名称</th>
<th>价格</th>
<th>库存</th>
<th>操作</th>
</tr>
<c:forEach var="product" items="${products}">
<tr>
<td>${product.name}</td>
<td>${product.price}</td>
<td>${product.stock}</td>
<td><a href="addCart.jsp?id=${product.id}">加入购物车</a></td>
</tr>
</c:forEach>
</table>
</body>
</html>
```
其中,${products}表示商品列表,每个商品都有一个id、name、price和stock属性,点击“加入购物车”按钮时,会跳转到addCart.jsp页面,并且将对应的商品id作为参数传递过去。
接下来,我们来实现addCart.jsp页面的逻辑,将选中的商品添加到购物车中。假设购物车中的数据存储在一个名为cart的session中,我们可以使用以下代码来实现:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
// 获取商品id
String productId = request.getParameter("id");
// 从数据库中查询商品信息
// ...
// 获取购物车中的商品列表
List<Product> cart = (List<Product>) session.getAttribute("cart");
if (cart == null) {
cart = new ArrayList<Product>();
session.setAttribute("cart", cart);
}
// 将选中的商品添加到购物车中
for (Product product : cart) {
if (product.getId().equals(productId)) {
product.setQuantity(product.getQuantity() + 1);
break;
}
}
Product product = new Product();
product.setId(productId);
product.setName("商品名称");
product.setPrice(100.0);
product.setStock(10);
product.setQuantity(1);
cart.add(product);
%>
商品已添加到购物车中!
```
在这个例子中,我们首先通过request.getParameter("id")方法获取商品id,然后从数据库中查询商品的详细信息。接着,我们从session中获取购物车列表,如果购物车列表为空,则创建一个新的空列表,并将其存储在session中。然后,我们遍历购物车列表,判断选中的商品是否已经存在于购物车中,如果存在,则将其数量加1,否则,创建一个新的商品对象,并将其添加到购物车中。
最后,我们输出一条信息,告诉用户商品已经成功添加到购物车中。
这就是一个简单的jsp+MySQL实现加入购物车的例子,希望能对你有所帮助。
相关推荐
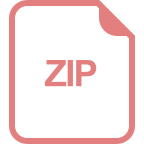
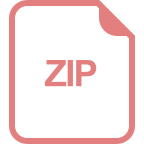














